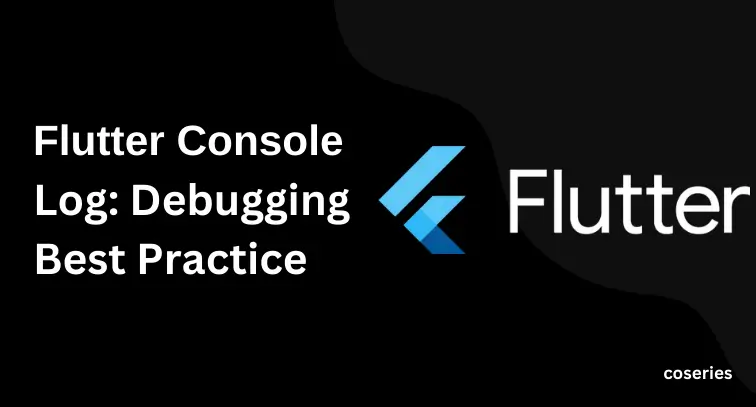
Debugging is a crucial part of the development process, and for Flutter developers, effectively logging into the console is key to diagnosing and fixing issues quickly. This article explores best practices for using the Flutter console log, helping you streamline your debugging process and improve the quality of your applications.
Table of Contents
Flutter Console Log Best Practice
Understanding Flutter Console Logging
Logging is an essential tool for developers to understand what their application is doing at any given moment. In Flutter, there are several ways to log messages to the console:
- print(): The most basic method for logging.
- debugPrint(): A wrapper around print() that throttles the output to avoid dropping log lines.
- developer.log(): A more advanced logging function that allows for more detailed and structured logging.
Each of these methods serves different purposes and knowing when to use each can make your debugging process more efficient.
Using print()
for Simple Logging
The print()
function is the most straightforward way to flutter console log messages in Flutter. It’s useful for quick, simple messages:
void main() {
String message = 'Hello, Flutter!';
print(message); // Outputs "Hello, Flutter!" to the console
}
However, print()
is not ideal for logging in production environments due to its simplicity and lack of control over the output.
Throttling Logs with debugPrint()
When logging large amounts of data, especially in Android, the system might drop some log lines. To avoid this, use debugPrint()
, which throttles the output:
import 'package:flutter/foundation.dart';
void main() {
debugPrint('This is a debug message that won't be dropped.');
}
Structured Logging with developer.log()
For more detailed logging, developer.log()
from the dart:developer
package is recommended. It allows you to add more context to your flutter console log messages:
import 'dart:developer' as developer;
void performAction() {
try {
// Code that might throw an exception
} catch (e, stackTrace) {
developer.log('An error occurred: $e', error: stackTrace, name: 'my.app.error');
}
}
This method is beneficial when you need to log errors along with their stack traces and categorize log messages.
Custom Logging Solutions
Creating a custom logging solution can give you more control over how your logs are handled. For instance, you can create a custom logger that formats messages and includes timestamps:
class CustomLogger {
void log(String message, {String level = 'INFO'}) {
final formattedMessage = _formatMessage(level, message);
print(formattedMessage);
}
String _formatMessage(String level, String message) {
return '${DateTime.now()} [$level] $message';
}
}
void main() {
CustomLogger logger = CustomLogger();
logger.log('Application started');
}
This approach allows you to tailor the logging to fit your needs, whether for debugging or monitoring purposes.
Conditional Logging Based on Environment
It’s essential to differentiate between logs for debugging and production. Use assertions and conditional logging to manage this:
void main() {
bool isDebug = false;
assert(isDebug = true); // This line will only execute in debug mode.
if (isDebug) {
print('Debug mode is enabled');
}
}
This ensures that certain logs are only present during development, preventing unnecessary log clutter in production.
Advanced Debugging Techniques
In addition to console logging, Flutter provides other tools for debugging:
- debugDumpApp(): Dumps the widget tree to the console.
- debugDumpRenderTree(): Dumps the render tree.
- debugDumpLayerTree(): Dumps the layer tree.
- debugDumpSemanticsTree(): Dumps the semantics tree.
These functions are useful for visualizing the state of your application and identifying layout or rendering issues.
Example: debugDumpApp
import 'package:flutter/material.dart';
void main() {
runApp(
const MaterialApp(
home: AppHome(),
),
);
}
class AppHome extends StatelessWidget {
const AppHome({super.key});
@override
Widget build(BuildContext context) {
return Material(
child: Center(
child: TextButton(
onPressed: () {
debugDumpApp();
},
child: const Text('Dump Widget Tree'),
),
),
);
}
}
Example: Dumping the Render Tree
import 'package:flutter/material.dart';
void main() {
runApp(
const MaterialApp(
home: AppHome(),
),
);
}
class AppHome extends StatelessWidget {
const AppHome({super.key});
@override
Widget build(BuildContext context) {
return Material(
child: Center(
child: TextButton(
onPressed: () {
debugDumpRenderTree();
},
child: const Text('Dump Render Tree'),
),
),
);
}
}
Example: Dumping the Layer Tree
import 'package:flutter/material.dart';
void main() {
runApp(
const MaterialApp(
home: AppHome(),
),
);
}
class AppHome extends StatelessWidget {
const AppHome({super.key});
@override
Widget build(BuildContext context) {
return Material(
child: Center(
child: TextButton(
onPressed: () {
debugDumpLayerTree();
},
child: const Text('Dump Layer Tree'),
),
),
);
}
}
Example: debugDumpSemanticsTree
import 'package:flutter/material.dart';
void main() {
runApp(
const MaterialApp(
home: AppHome(),
),
);
}
class AppHome extends StatelessWidget {
const AppHome({super.key});
@override
Widget build(BuildContext context) {
return Material(
child: Center(
child: TextButton(
onPressed: () {
debugDumpSemanticsTree();
},
child: const Text('Dump Semantics Tree'),
),
),
);
}
}
Conclusion
Effective logging is a cornerstone of efficient debugging. By leveraging the various logging methods available in Flutter, from basic print()
statements to more advanced developer.log()
you can ensure that your debugging process is both thorough and efficient by combining them with custom logging solutions and environment-based conditional logging. Utilize the advanced debugging techniques provided by Flutter to gain deeper insights into your application’s behavior and resolve issues more quickly.
By adopting these best practices for the Flutter console log, you can enhance your development workflow, making diagnosing and fixing problems easier, and ultimately delivering more robust and reliable applications.
Read More:
How to Add Container Border in Flutter in 2024
Inspired by: docs.flutter.dev