In this article, i will guide how to use properly to add container border in Flutter. Flutter Container is the one of the important widget to serve this kind of flutter container border task. Containers are the foundation of building user interfaces (UI) in Flutter. They act as versatile building blocks for various UI elements. Often, you’ll want to add borders to these containers to create visual distinction, separate content areas, or mimic real-world elements like buttons and cards. This guide dives deep into Container Border in Flutter, providing step-by-step instructions and code examples to achieve different border styles.
Add Container Border in Flutter
Let’s start with the fundamentals. Here’s how to add a simple border around your container using BoxDecoration:
Container(
width: 200,
height: 200,
decoration: BoxDecoration(
border: Border.all(
width: 5,
color: Colors.teal,
),
),
),
This code creates a container with a width of 200 pixels, a height of 200 pixels, and a solid teal border with a width of 5 pixels. You can customize the width and color of the border to match your design preferences.
Output:
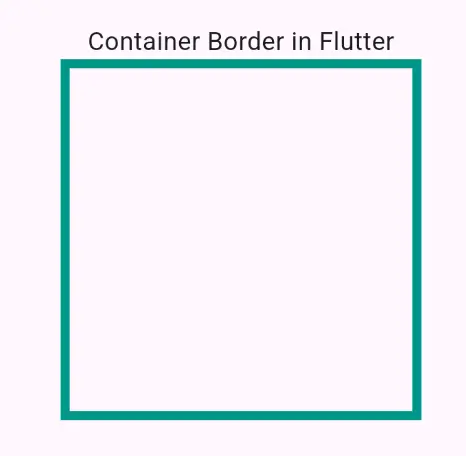
Add Flutter Container Border for Specific Side
For more control, you can define borders for individual sides of the container. Code for Flutter Container Border for Specific Side using BorderSide:
Container(
width: 200,
height: 200,
decoration: const BoxDecoration(
border: Border(
left: BorderSide(
color: Colors.teal,
width: 6.0,
),
top: BorderSide(
color: Colors.tealAccent,
width: 10.0,
),
),
),
),
This code applies a teal border with a width of 6 pixels to the left side of the container and a teal accent border with a width of 10 pixels to the top side. Feel free to experiment with different colors and thicknesses for a unique look.
Output:
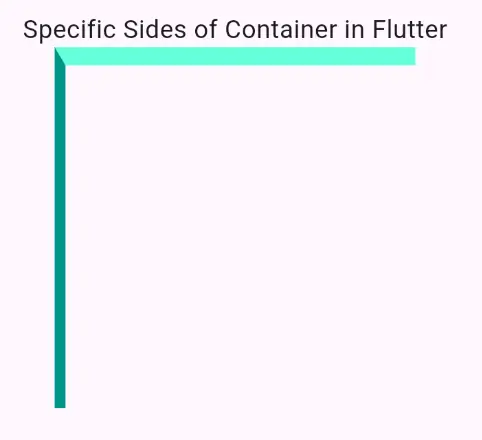
Add Flutter Border for Symmetrical Side of Container
If you want the same border style on opposite sides of the container, Flutter offers a convenient shortcut. Code for Border of Symmetrical Side of Container in Flutter using Border.symmetric:
Container(
width: 200,
height: 200,
decoration: const BoxDecoration(
border: Border.symmetric(
horizontal: BorderSide(
color: Colors.blueGrey,
width: 2,
),
vertical: BorderSide(
color: Colors.pink,
width: 7,
),
),
),
),
Here, we define symmetrical borders using Border.symmetric
. The horizontal
property sets a blue-grey border with a width of 2 pixels for both the left and right sides, while the vertical
property creates a pink border with a width of 7 pixels for the top and bottom sides.
Output:
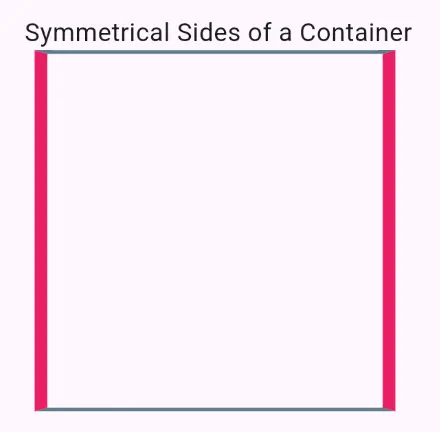
By mastering these techniques, you can create a wide variety of Container Border in Flutter styles to enhance your app’s visual appeal and user experience. Remember to experiment and explore different color combinations and border widths to achieve your desired design goals with container border in flutter!
If you have any question regarding container border in flutter, please leave a comment or book a call from top sections.