Flutter Substrings are essential in displaying and managing text in your Flutter applications. One of the common operations you may need is manipulating parts of a string, which can be efficiently achieved using substring techniques. This article will explore various ways to work with substrings in Flutter, including extracting, modifying, and formatting strings.
In this comprehensive guide, we’ll dive deep into the concept of Flutter substrings, highlight best practices, and provide hands-on examples to optimize your Flutter development.
Table of Contents
Flutter Substrings
1. Introduction to Flutter Strings
In Flutter, strings are immutable, meaning their content cannot be changed once created. However, there are various operations you can perform to manipulate or extract parts of a string. Flutter’s String class provides built-in methods for efficient string manipulation, making it easy to handle tasks like searching, trimming, replacing, and more.
Example:
String greeting = "Hello, Flutter!";
In the above example, the greeting is a simple string variable in Dart (the language used by Flutter). You can manipulate or extract specific parts of this string using substring techniques.
2. Understanding Flutter Substrings:
A substring is simply a portion of a string. In Dart (and hence in Flutter), you can use the substring method to extract a portion of a string between specific indices. This method requires two parameters:
- startIndex: The index from where to start the extraction.
- endIndex (optional): The index at which the extraction should stop (exclusive).
The substring method is zero-indexed, meaning the first character is at index 0.
Syntax:
String substring(int startIndex, [int? endIndex]);
3. Extracting Substrings
Let’s dive into how you can extract substrings in Flutter.
Example 1: Extracting with only startIndex
void main() {
 String message = "Welcome to Flutter!";
 String part = message.substring(11);
 print(part);Â
}
Output:
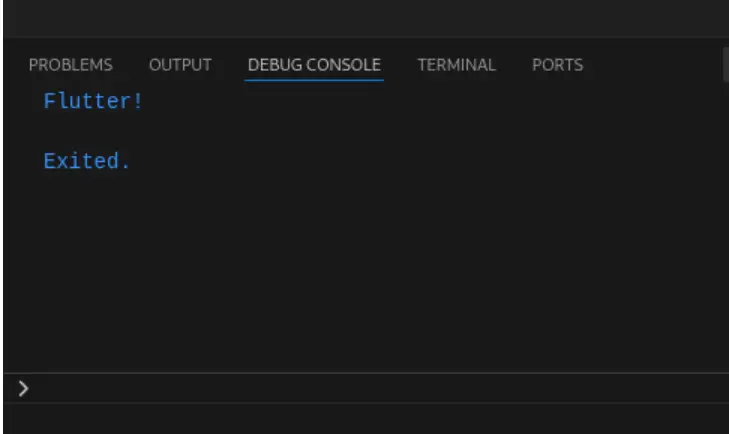
In this example, we start from index 11, and since no endIndex is provided, it extracts everything from index 11 to the end of the string.
Example 2: Extracting with both startIndex and endIndex
void main() {
 String message = "Welcome to Flutter!";
 String part = message.substring(0, 7);
 print(part);
}
Here, the substring method extracts from index 0 to index 7 (exclusive), giving us the word “Welcome”.
Output:
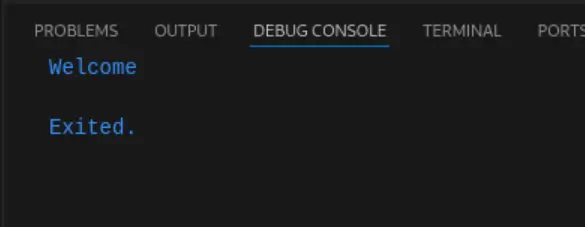
4. Using Substring for String Manipulation
Substrings are helpful for various string manipulation tasks, such as trimming specific parts, slicing user input, or formatting strings.
Example 3: Using substring for URL trimming
void main() {
 String url = "https://www.example.com/page";
 String domain = url.substring(8, 23);
 print(domain); // Output: www.example.com
}
This trims the domain from a full URL by extracting the substring between specific indices.
Output:
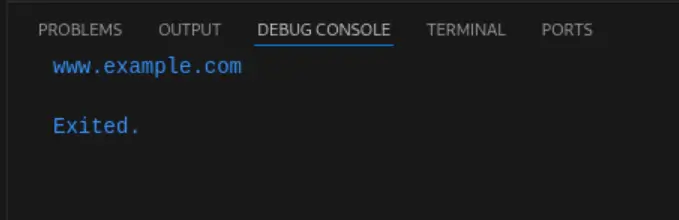
5. Common Use Cases of Flutter SubStrings
1. User Input Validation
When handling user input, you may want to extract certain parts (e.g., country code from a phone number).
void main() {
 String phoneNumber = "+1-555-555-5555";
 String countryCode = phoneNumber.substring(0, 2);
 print(countryCode); // Output: +1
}
Output:
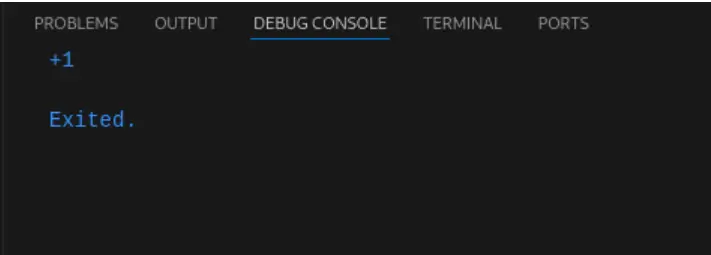
2. Truncating Long Text
You might need to shorten a string to display a preview in your app.
void main() {
 String description = "This is a long description that needs truncating";
 String truncated = "${description.substring(0, 20)}...";
 print(truncated); // Output: This is a long desc...
}
Output:
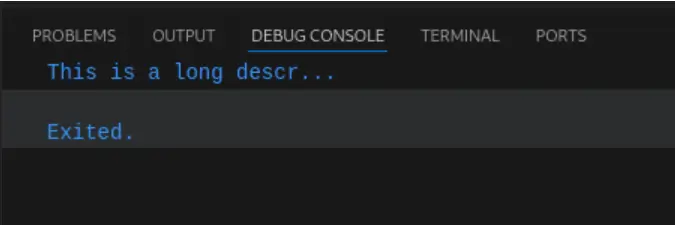
3. Extracting Date or Time
Extracting specific parts of a date-time string can be useful for formatting.
void main() {
 String dateTime = "2024-10-05 14:30:00";
 String date = dateTime.substring(0, 10);
 print(date); // Output: 2024-10-05
}
Output:
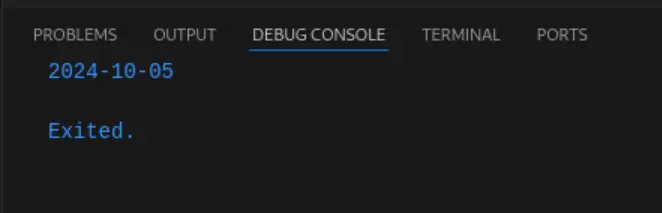
6. Error Handling with Substrings
Handling potential errors is crucial when working with substrings. If you try to extract a substring using an index that is out of bounds, Dart will throw a RangeError.
Example:
void main() {
 String message = "Flutter is fun!";
 try {
   String part = message.substring(0, 20);
   print(part);
 } catch (e) {
   print("Error: $e");
 }
}
To avoid this, always ensure that your indices are within the valid range of the string.
Output:
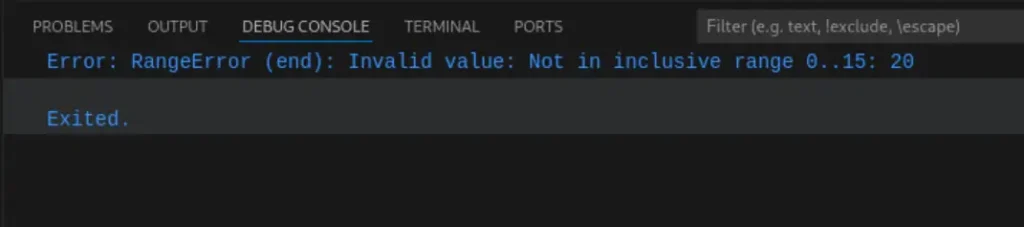
7. Best Practices for Efficient String Manipulation
Index Validations: Always validate the start and end indices when working with substring.
Avoid Overuse: While substrings are powerful, overusing them can make your code less readable. Use meaningful variable names to store extracted parts.
Use Regular Expressions: Regular expressions (RegExp) might be more efficient and expressive than substring operations for complex string manipulations.
8. Conclusion
Substrings are a fundamental part of string manipulation in Flutter, allowing you to extract and modify parts of a string efficiently. With the substring method and other string operations, you can handle user input, manipulate text, and format data as required in your Flutter applications.
By following best practices and understanding the common use cases, you can optimize the performance and readability of your code while working with strings in Flutter.
With the insights and examples provided, you’re well-equipped to start using Flutter substrings effectively in your apps.
You can read also: Enhance Your App’s User Experience With Full Screen In Flutter