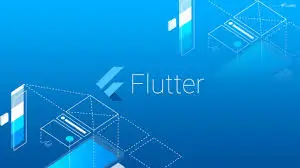
Flutter How to Get Assets Image Path: Unlock the Ultimate Guide
in Flutter on October 16, 2024by Kawser MiahFlutter How to Get Assets Image Path is essential when building a Flutter app, as you often need to include and display images from your assets directory. Flutter provides a straightforward way to handle images, but beginners sometimes struggle with correctly accessing the image path. In this article, we’ll explore Flutter How to Get Assets Image Path easily and ensure that your images load without issues. We will cover step-by-step instructions along with proper code examples and explanations.
Table of Contents
Flutter How to Get Assets Image Path
Understanding the Basics of Assets in Flutter
Assets in Flutter include any non-code files, such as images, fonts, or JSON files, that are bundled with your app and can be used at runtime. The key to using these assets is correctly defining them in the pubspec.yaml
file and using them in your code with the correct path. Let’s dive into Flutter How to Get Assets Image Path efficiently.
Step 1: Defining Assets in pubspec.yaml
To get started, you must define the images in the pubspec.yaml
file. Flutter uses this file to locate your assets. If you skip this step, you will face issues such as images not loading in your app, making the Flutter How to Get Assets Image Path process crucial.
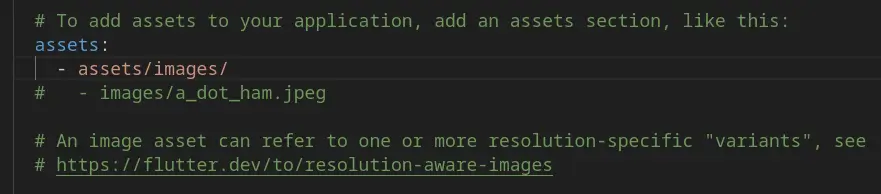
Step 2: Accessing Assets Image Path in Code
Now that the images are defined in pubspec.yaml
, we can access them in our code. The Flutter Image widget is typically used to display images. Here’s how to access Flutter How to Get Assets Image Path in your code:
import 'package:flutter/material.dart';
class HomePage extends StatelessWidget {
const HomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: Colors.teal[50],
appBar: AppBar(
centerTitle: true,
backgroundColor: Colors.teal[100],
title: const Text('How to Get Assets Image Path in Flutter'),
),
body: Padding(
padding: const EdgeInsets.all(28.0),
child: Center(
child: Column(
children: [
const Text(
'Using Image.asset()',
style: TextStyle(
fontSize: 20,
fontWeight: FontWeight.bold,
),
),
Image.asset(
'assets/images/canda.jpg',
height: 380,
fit: BoxFit.fitHeight,
),
],
),
),
),
);
}
}
This simple code snippet shows how to easily use the Flutter How to Get Assets Image Path. We access the images using the Image.asset()
method, providing the relative path of the image as defined in pubspec.yaml
.
Step 3: Understanding Image Path in Flutter
To ensure your images are properly loaded, the Flutter How to Get Assets Image Path process must be correctly implemented in both your pubspec.yaml
file and code. Always check that:
- The assets path in
pubspec.yaml
matches the actual folder structure. - Images are placed in the correct directory (e.g., assets/images/).
- The image file names are accurate (case-sensitive).
Output of the Code:
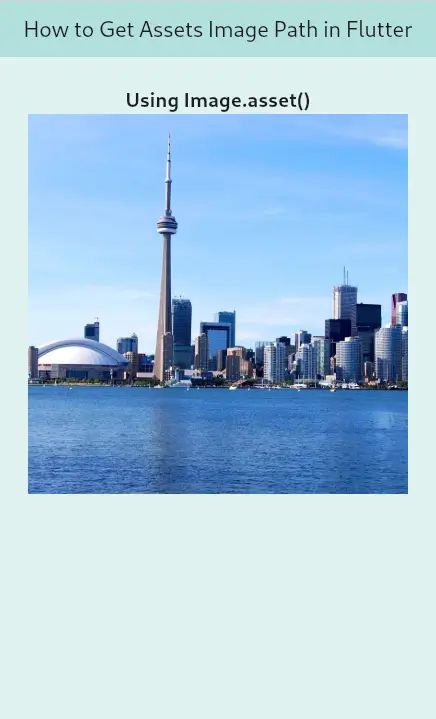
Step 4: Handling Errors with Image Path
Sometimes, even after defining assets properly, images may not load, especially if there are mistakes in the path or pubspec.yaml
file. If you encounter errors like “Unable to load an asset,” make sure your Flutter How to Get Assets Image Path is correct.
Here’s an example of incorrect asset path usage:
Image.asset('assets/wrong_folder/phone.png'); // Wrong path
Output:
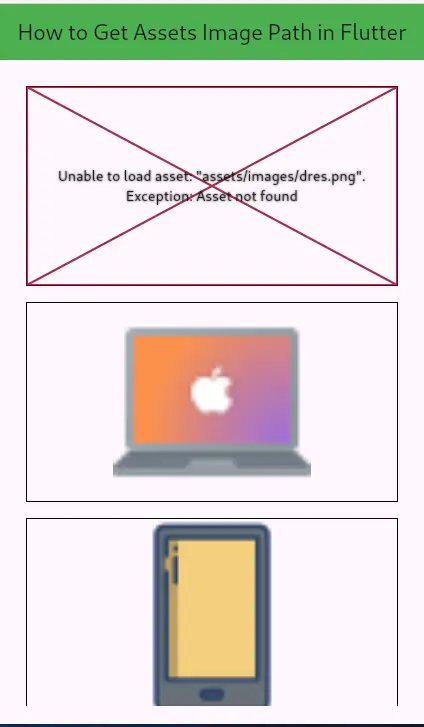
This will throw an error because the image is not located in the specified folder. Ensure you use the correct path when accessing Flutter How to Get Assets Image Path.
Step 5: Using AssetImage for More Control
In some cases, you might want more control over the image-loading process, such as caching or preloading. Flutter provides the AssetImage
class for these purposes. Let’s see how we can utilize it to work with Flutter How to Get Assets Image Path:
Image(
height: 400,
width: 400,
fit: BoxFit.cover,
image: AssetImage('assets/images/japan.jpg'),
),
By using AssetImage
, you get more control over how the image is loaded and cached. This method can be useful when you need to manipulate images further while still following Flutter How to Get Assets Image Path.
Output:
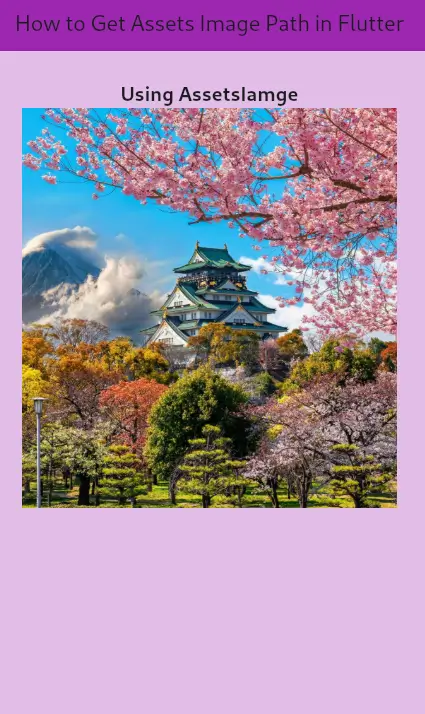
Conclusion
Getting the correct Flutter How to Get Assets Image Path is crucial to displaying images properly in your app. The process involves defining the assets in pubspec.yaml
and access them in your code using Image.asset()
or AssetImage
. By following these steps, you can ensure that your images load without any issues. Whenever you face problems with images not loading, double-check your asset path, pubspec.yaml
file, and folder structure.
Mastering Flutter How to Get Assets Image Path will save you a lot of trouble and make your app development process smoother. Now, you’re ready to incorporate images seamlessly into your Flutter app!
You can read also: A Professional Guide For Dynamic TabBar In Flutter