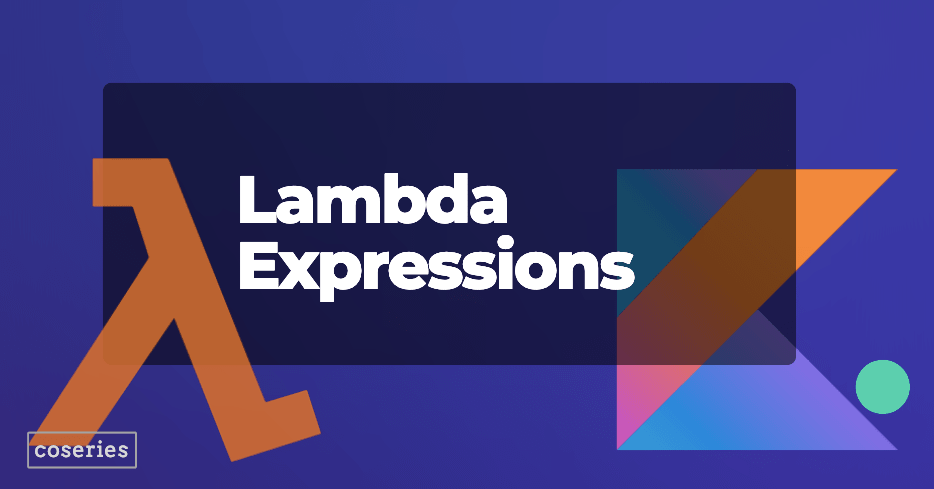
Kotlin Lambda Functions & Higher Order Functions in 2024?
in Kotlin, Android on May 8, 2024by Mafujul IslamKotlin Lambda Functions is one of the most powerful tools in Kotlin. There are so many programming languages who has the Lambda Expression tool. Such as: Java, C#, C++, Python, etc. Like these programming languages, Kotlin also has this Lambda Expressions feature.
In this article, we will be learning what the Lambda Expression is and some other topics related to Lambda Expressions.
What is Kotlin Lambda Functions?
The concept of the Lambda comes from Lambda Calculus. This Lambda Calculus is a formal system used in computer science where different kinds of computations could be represent with variables.
In simple words, Kotlin Lambda Functions is a simplified representation of a function. And this function could be passed as a parameter to any Higher Order Function and could be returned from a function as a value. This simplified function also could be represented by a variable.
Kotlin Lambda Functions is an anonymous function. It does mean, this function has no name.
fun sum(x: Int, y: Int): Int{ return x + y }
This is a very simple regular function, starts with fun
keyword. It has a name (sum
), two parameter (x: Int, y: Int
), and a body with curly braces. The return type of this function is Int.
The Kotlin Lambda Functions is similar to this, but very simplified. Let’s have a look on a Lambda Function, which is similar to the above’s regular function.
val sum: (Int, Int) -> Int = { x: Int, y: Int -> x + y }
This is the full syntactic form of the Kotlin Lambda Functions and Expressions and its task is similar to the previous regular function. Let’s see its different parts:

Lambda Expression is always surrounded by curly braces and the main Lambda Expression part here is after the equal (=) sign. Inside the curly braces, it has two parameter. And the body part goes after the -> sign. If the return type of the lambda is not Unit, then the last expression (or last statement) inside the body will be acted as the return value (here, x + y
is the return value, and its type is Int).
The lambda function is assigned to variable sum
. Here, the type portion ((Int, Int) -> Int
) shows, this function requires two integer type parameter and the return value of this function will also be integer. But as the Kotlin language is Type Inferred, we can ignore the type part of the lambda expressions.
After ignoring the type part, the simplified lambda expression is:
val sum = { x: Int, y: Int -> x + y }
Here, the portion enclosed with curly braces is the main Lambda Expression. And we assign it to the variable sum
. Now we can use this function by sum
and we also can pass it as a parameter to another function.
What is Higher-Order Function?
A function which can take another function as parameters or can return another function is called Higher-Order Function.
Let’s have an example:
fun printNames(names: ArrayList<String>, printer: (String) -> Unit){ for (name in names){ printer(name) } }
This is a function named printNames
and this function will take two parameters, one is array list of string (names: ArrayList<String>
) and another one is a function (printer: (String) -> Unit)
). Here, “printer: (String) -> Unit)
” is the definition of a Kotlin Lambda Functions. The name of the function is printer
and it takes a string as parameter and return a Unit (Unit is a keyword that says, this function does not return anything). Instead of Unit, we could use String for returning string, or Int for returning integer or other types.
As this function is taking another function as parameter, so it is a Higher-Order Function.
The way to use this function is as follows:
val names = arrayListOf("coseries", "Mafs", "Sho", "Mafu") val myLambda = {name: String -> println(name)} printNames(names, myLambda)
We can simplify it more by putting the lambda function inside the printNames function calling parentheses.
val names = arrayListOf("coseries", "Mafs", "Sho", "Mafu") printNames(names, {name: String -> println(name)})
If the Kotlin Lambda Functions is the last parameter of a function or only parameter of a function, then in Kotlin, we can put it outside the parenthesis. Here is how we can do it.
val names = arrayListOf("coseries", "Mafs", "Sho", "Mafu") printNames(names) { name: String -> println(name)}
Some Common Higher-Order Functions (HOFs)
Higher-Order Function are frequently used when we deal with Collections. In collections, there are several built-in functions we are using very often. Let’s explore some very common Higher-Order Functions which are being used frequently.
1. forEach
forEach is a Higher-Order Function which takes a lambda as parameter and this HOF applied to a collection. This function is an existing function shipped with Kotlin. The task of this function is to iterate the collection from position 0 to n, and in each iteration, the function will return an item with ascending order.
val names = arrayListOf("coseries", "Mafs", "Sho", "Mafu") names.forEach { name: String -> println(name) }
As the Kotlin Lambda Functions (used as parameter of forEach function) has only one parameter, we can refer that parameter by “it
” keyword.
val names = arrayListOf("coseries", "Mafs", "Sho", "Mafu") names.forEach { println(it) }
2. filter
This is another frequently used HOF which takes a boolean parameter. This function returns a collection with the filtered items.
val names = arrayListOf("coseries", "Mafs", "Sho", "Mafu") names.filter { it[0] == 'M' }.forEach { println(it) }
Output of the above code is as follow:
Mafs Mafu
The filter
function will return a filtered collection with items “Mafs” and “Mafu”. Because, these two items contain “M” at their 0th position. Finally, forEach
HOF is applied to the filtered collection to print the items.
3. sortedBy
For sorting a collection, we use this HOF
val names = arrayListOf("coseries", "Mafs", "Sho", "Mafu") names.sortedBy { it.length }.forEach { println(it) }
Here, the collection names will be sorted by the lengths of the items. Here the length of the item “Sho” is 3 and the length of the item “coseries” is 8. So the first item of the new collection will be “Sho” and the last item of the collection will be “coseries”.
Output of the above code will be like this:
Sho Mafs Mafu coseries
In addition to these, there are so many Higher-Order Functions applied in collections we can find by just putting a dot after the collection (IDE will suggest us). Other than this, we also can make this type of HOF function.