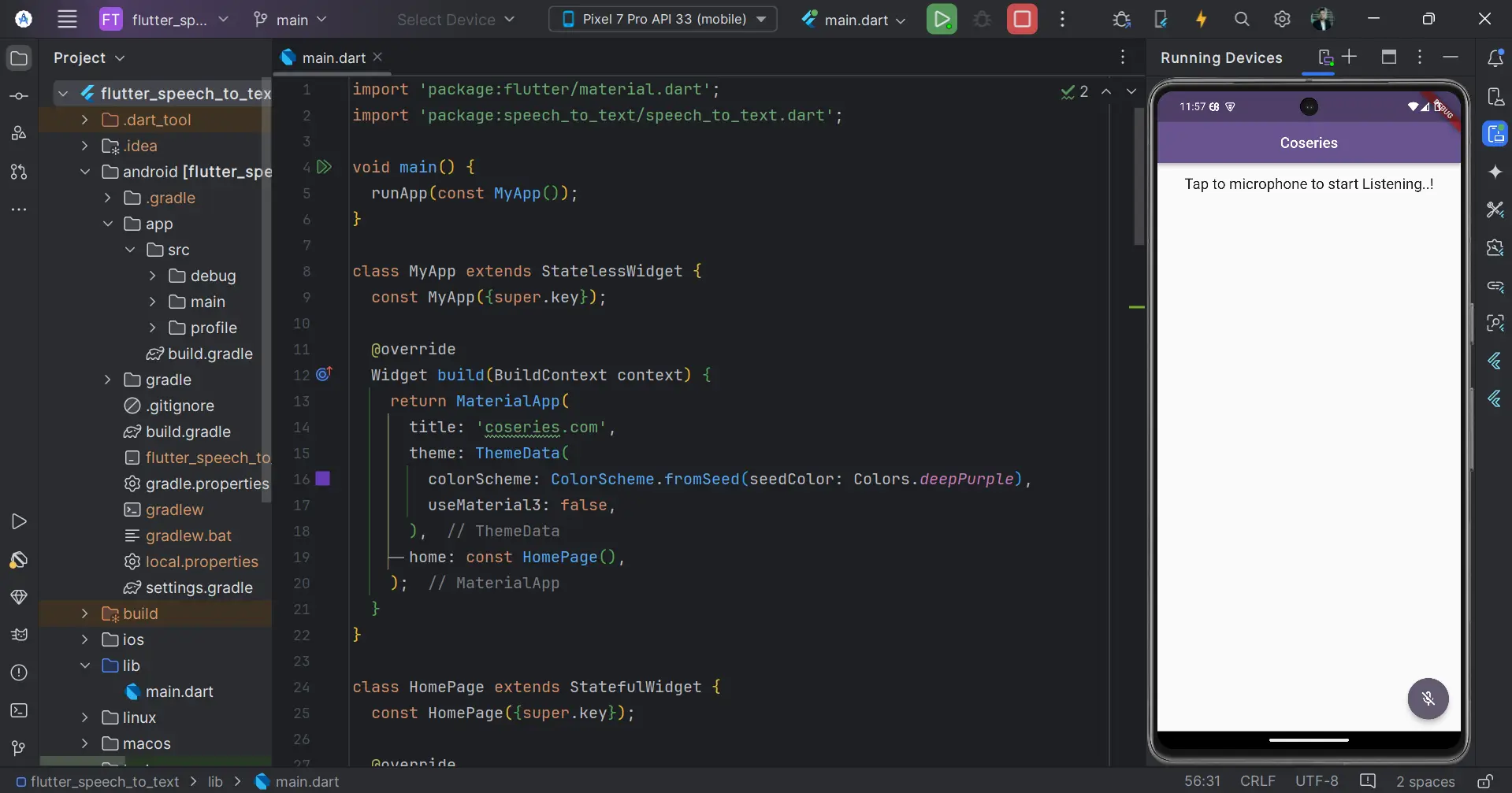
Flutter Speech to Text: Enhance Your App’s Functionality
in Flutter on August 26, 2024by Kawser MiahThe Flutter Speech to Text capability, enabled by the speech_to_text package, provides developers with a straightforward way to add speech recognition functionality to their Flutter apps. This package not only simplifies the process of converting speech to text but also empowers developers to create innovative applications that respond to user voice inputs. In this article, we’ll explore how to leverage this package to build voice-enabled Flutter apps using Flutter Speech to Text.
Table of Contents
Flutter Speech to Text
1. Understanding the Speech_to_Text Package
1.1. What is the speech_to_text package?
The speech_to_text package is a Flutter plugin that allows developers to add Flutter Speech to Text capabilities to their apps. This package can convert spoken words into text in real time, enabling a variety of voice-controlled features. It supports both Android and iOS platforms, making it a versatile choice for cross-platform Flutter applications.
1.1.1. Capabilities:
- Real-time Flutter Speech to Text recognition.
- Continuous listening mode to capture long speech inputs.
- Access to recognized words as they are spoken.
- Support for multiple languages and dialects.
1.1.2. Limitations:
- Requires a stable internet connection for accurate Flutter Speech to Text recognition.
- The quality of recognition may vary depending on background noise and microphone quality.
1.2. Why use speech_to_text in your Flutter app?
Integrating Flutter Speech to Text functionality into your app can open up a range of possibilities:
- Voice Commands: Allow users to control the app using their voice, making it more interactive and accessible.
- Transcriptions: Automatically transcribe spoken words into text for features like note-taking, messaging, or captions.
- Accessibility: Improve the app’s accessibility for users with disabilities, enabling them to interact with the app without needing to type or touch the screen.
2. Installation and Setup
2.1. Adding the Dependency
To get started, you’ll need to add the speech_to_text package to your Flutter project. Open your pubspec.yaml file and add the following dependency:
dependencies:
speech_to_text: ^7.0.0 //Replace with the latest version
2.2. Configuring platform-specific permissions
For Flutter Speech to Text recognition to work, your app needs permission to access the microphone. The configuration differs slightly for Android and iOS:
Android:
Add the record audio permission to your AndroidManifest.xml file, located in <project root>/android/app/src/main/AndroidManifest.xml
.
<uses-permission android:name="android.permission.RECORD_AUDIO"/>
<uses-permission android:name="android.permission.INTERNET"/>
<uses-permission android:name="android.permission.BLUETOOTH"/>
<uses-permission android:name="android.permission.BLUETOOTH_ADMIN"/>
<uses-permission android:name="android.permission.BLUETOOTH_CONNECT"/>
iOS:
and add the following key to Info.plist file for IOS.
NSSpeechRecognitionUsageDescription
NSMicrophoneUsageDescription
3. Implementing Speech Recognition
Here’s an example of how to use the Flutter Speech to Text to download and cache an image:
final SpeechToText speechToText = SpeechToText();
bool _speechEnable = false;
String wordSpoken = "";
double confidenceLevel = 0;
@override
void initState() {
// TODO: implement initState
super.initState();
initSpeech();
}
void initSpeech() async {
_speechEnable = await speechToText.initialize();
setState(() {});
}
void startListening() async {
await speechToText.listen(onResult: onSpeechResult);
setState(() {
confidenceLevel = 0;
});
}
void stopListening() async {
await speechToText.stop();
setState(() {});
}
void onSpeechResult(result) async {
setState(() {
wordSpoken = "${result.recognizedWords}";
confidenceLevel = result.confidence;
});
}
Output:
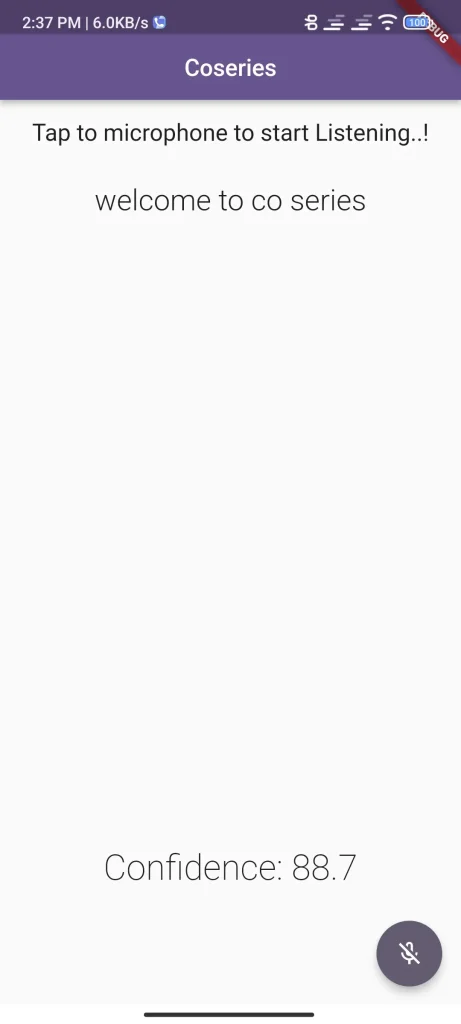
4. Explanation
4.1. Variable Declarations
- SpeechToText speechToText: This is an instance of the SpeechToText class, which is responsible for handling all speech recognition functionalities.
- _speechEnable: A boolean variable that indicates whether speech recognition is enabled on the device.
- wordSpoken: A string that stores the text recognized from the user’s speech.
- confidenceLevel: A double that represents the confidence level of the recognized words, indicating how accurate the recognition is.
4.2. initState Method
- initState: This method is called when the widget is first created. It’s used to initialize any state-related data.
- initSpeech: This function is called within initState to initialize speech recognition.
4.3. initSpeech Method
- initSpeech: This asynchronous function initializes the SpeechToText instance.
- speechToText.initialize(): This method attempts to initialize the speech recognizer. It returns true if the device supports speech recognition.
- _speechEnable: The result of the initialization is stored in this variable.
- setState(() {}): This triggers a UI update to reflect any changes, such as enabling or disabling certain UI elements based on _speechEnable.
4.4. startListening Method
- startListening: This method starts listening for speech.
- speechToText.listen(onResult: onSpeechResult): This begins the speech recognition process and assigns the onSpeechResult method as the callback to handle the recognized speech.
- confidenceLevel = 0: Resets the confidence level when a new listening session starts.
- setState(() {}): Updates the UI to reflect the changes.
4.5. stopListening Method
- stopListening: This method stops the speech recognition process.
- speechToText.stop(): Stops the current listening session.
- setState(() {}): Updates the UI after stopping the speech recognition.
4.6. onSpeechResult Method
- stopListening: This method stops the speech recognition process.
- speechToText.stop(): Stops the current listening session.
- setState(() {}): Updates the UI after stopping the speech recognition.
- onSpeechResult Method
- onSpeechResult: This method is triggered whenever the speech_to_text package recognizes speech.
- result.recognizedWords: The recognized speech is accessed through result.recognizedWords and stored in the wordSpoken variable.
- result.confidence: The confidence level of the recognized words is stored in confidenceLevel.
- setState(() {}): Updates the UI to display the recognized words and confidence level.
5. Common Issues with the Speech_to_Text Package
5.1. Speech Recognition Not Starting
Symptoms: The app fails to start Flutter Speech to Text recognition. The microphone icon doesn’t change state, and no speech is recognized.
Solution:
- Ensure that you’ve properly initialized the SpeechToText instance. Double-check the initialize() method and ensure it returns true.
- Verify that microphone permissions are correctly configured in AndroidManifest.xml (Android) and Info.plist (iOS).
- Make sure the device has a stable internet connection, as Flutter Speech to Text often requires online processing.
5.2. Speech Recognition Not Available on the Device
Symptoms: The app reports that Flutter Speech to Text is not available, even on devices that should support it.
Solution:
- Call hasPermission and initialize methods early in your app to check if the device supports Flutter Speech to Text.
- Ensure the device language settings match the languages supported by the speech_to_text package.
- If testing on an emulator, try using a physical device, as emulators may have limitations with microphone input and Flutter Speech to Text.
5.3. Poor Recognition Accuracy
Symptoms: The Flutter Speech to Text transcriptions are inaccurate or fail to capture speech correctly. Users complain about misrecognition, especially in noisy environments.
Solution:
- Test in a quiet environment to rule out background noise as a factor.
- Use external microphones or better-quality devices to improve Flutter Speech to Text recognition accuracy.
- Consider filtering out background noise programmatically before feeding the audio to the speech recognizer (although this might require additional packages or custom solutions).
- Check that the language and locale settings match the user’s speech pattern.
5.4. Speech Recognition Stops Unexpectedly
Symptoms: The app stops Flutter Speech to Text recognition after a short period, even though the user is still speaking. The onResult callback stops being called prematurely.
Solution:
- Increase the pauseFor and listenFor parameters in the listen() method to allow for longer Flutter Speech to Text input.
- Ensure that isFinal is being checked correctly in the onResult callback to handle ongoing Flutter Speech to Text recognition.
- Look into memory management if the app is terminating the speech recognizer unexpectedly, especially in resource-constrained environments.
5.5. Platform-Specific Behaviors
Symptoms: The app behaves differently on Android and iOS, with issues like varying Flutter Speech to Text accuracy or different timeout settings.
Solution:
- Recognize that the speech_to_text package may interact differently with Android and iOS APIs. Tailor your implementation to handle these platform-specific nuances.
- Adjust timeout and sensitivity settings according to the platform.
- Regularly test on both Android and iOS devices to ensure consistent Flutter Speech to Text behavior.
6. Full Code
import 'package:flutter/material.dart';
import 'package:speech_to_text/speech_to_text.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'coseries.com',
theme: ThemeData(
colorScheme: ColorScheme.fromSeed(seedColor: Colors.deepPurple),
useMaterial3: false,
),
home: const HomePage(),
);
}
}
class HomePage extends StatefulWidget {
const HomePage({super.key});
@override
State<HomePage> createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
final SpeechToText speechToText = SpeechToText();
bool _speechEnable = false;
String wordSpoken = "";
double confidenceLevel = 0;
@override
void initState() {
// TODO: implement initState
super.initState();
initSpeech();
}
void initSpeech() async {
_speechEnable = await speechToText.initialize();
setState(() {});
}
void startListening() async {
await speechToText.listen(onResult: onSpeechResult);
setState(() {
confidenceLevel = 0;
});
}
void stopListening() async {
await speechToText.stop();
setState(() {});
}
void onSpeechResult(result) async {
setState(() {
wordSpoken = "${result.recognizedWords}";
confidenceLevel = result.confidence;
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text("Coseries"),
centerTitle: true,
),
body: Center(
child: Column(
children: [
Container(
padding: const EdgeInsets.all(16),
child: Text(
speechToText.isListening
? "Listening..!"
: _speechEnable
? "Tap to microphone to start Listening..!"
: "Speech not available",
style: const TextStyle(fontSize: 20),
),
),
Expanded(
child: Container(
padding: const EdgeInsets.all(16),
child: Text(
wordSpoken,
style:
const TextStyle(fontSize: 25, fontWeight: FontWeight.w300),
),
)),
if (speechToText.isNotListening && confidenceLevel > 0)
Padding(
padding: const EdgeInsets.only(bottom: 100.0),
child: Text(
"Confidence: ${(confidenceLevel * 100).toStringAsFixed(1)}",
style: const TextStyle(
fontSize: 30, fontWeight: FontWeight.w200),
),
)
],
),
),
floatingActionButton: FloatingActionButton(
onPressed: speechToText.isListening ? stopListening : startListening,
tooltip: "Listen",
child: Icon(speechToText.isNotListening
? Icons.mic_off_outlined
: Icons.mic_none_outlined),
),
);
}
}
Output:
7. Conclusion
Integrating the speech_to_text package into your Flutter app can significantly enhance the user experience by enabling voice interaction. Whether you’re building an accessibility feature or simply adding convenience, Flutter Speech to Text is a powerful tool. By following this guide, you can implement Flutter Speech to Text functionality in your own projects, opening up new possibilities for user engagement.
8. Additional Resources
8.1. Links
You can read also: Efficient data caching for your flutter Application with CacheManager