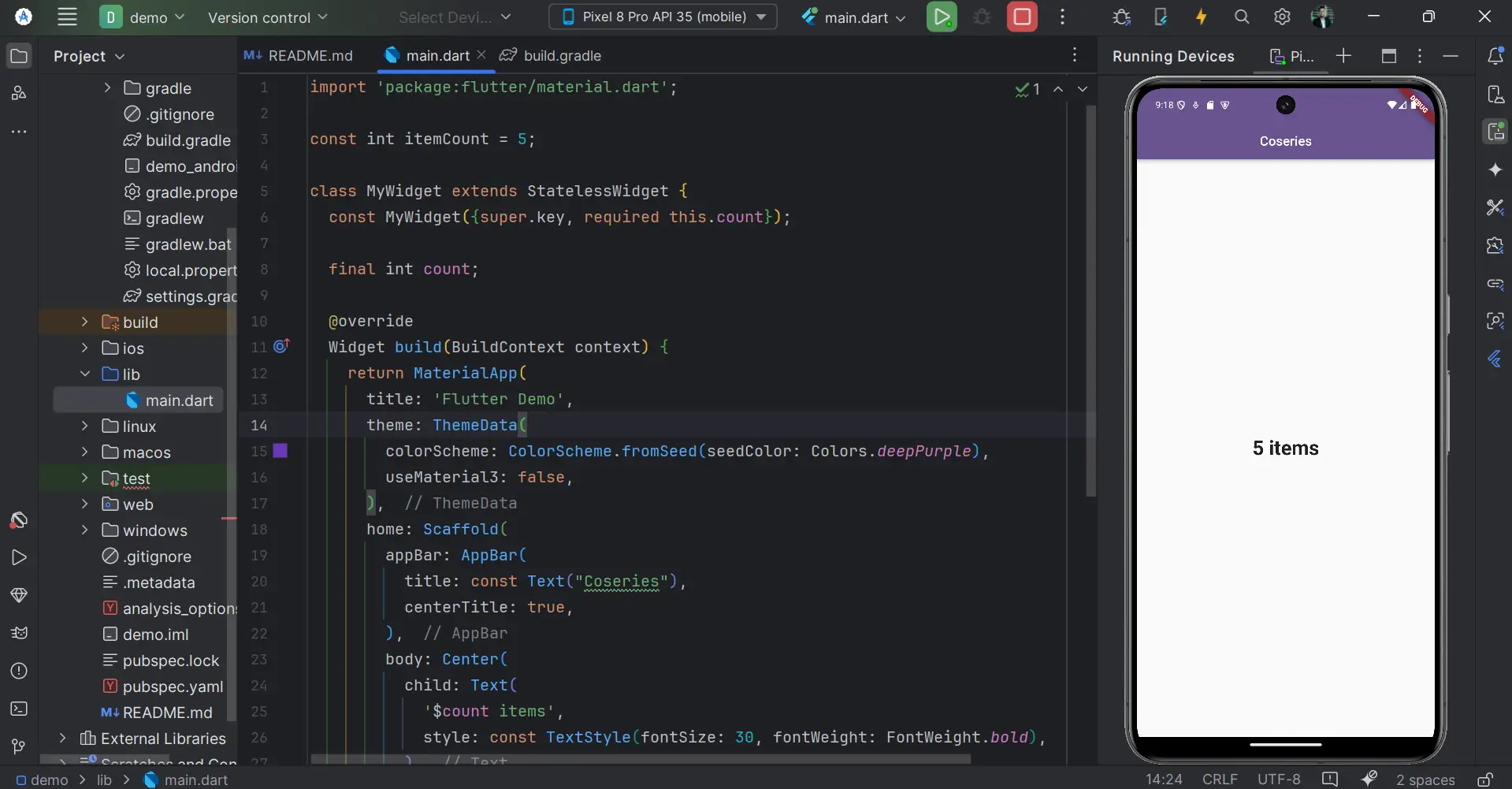
Flutter Invalid Constant Value Using Variable as Parameter: Resolving Variable Parameter Issues
in Flutter on November 26, 2024by Kawser MiahIn Flutter development, Flutter Invalid Constant Value Using Variable as Parameter is a frequent issue that developers encounter when working with constants in their applications. Constants in Flutter are valuable for creating efficient, stable applications by reducing unnecessary rebuilds and optimizing memory usage. Using the const keyword allows us to define compile-time constants in Flutter, ensuring that their values remain unchanged throughout the program’s lifecycle. However, this error often appears when trying to pass variables as parameters in places that expect constant values. Let’s dive into why the Flutter Invalid Constant Value Using Variable as Parameter error occurs and explore solutions to ensure your code runs smoothly.
Table of Contents
Flutter Invalid Constant Value Using Variable as Parameter
1.1 What is const in Flutter and When to Use It?
The const keyword signifies compile-time constants, which are values determined before the app runs. By using const, Flutter treats the objects as immutable and reusable, meaning it can optimize memory usage effectively. However, misuse can trigger the Flutter Invalid Constant Value Using Variable as Parameter error, especially when constants are misapplied to non-constant values.
1.2 Difference Between final and const
While both final and const ensure immutability, const is stricter. With const, all values must be known at compile time, while the final can be assigned at runtime. This difference is crucial when dealing with the Flutter Invalid Constant Value Using Variable as Parameter error, as it often emerges from attempting to use const where only final or runtime values would work.
2. Understanding the “Flutter Invalid Constant Value Using Variable as Parameter” Error
The Flutter Invalid Constant Value Using Variable as Parameter error occurs when a non-constant, like a variable or runtime data, is passed to a const widget. Flutter’s strict constant requirement doesn’t allow variables where a constant is expected, which is why this error frequently appears in code involving const constructors.
2.1 Why This Error Occurs
The Flutter Invalid Constant Value Using Variable as Parameter error arises when we attempt to pass a non-constant parameter to a widget with a const constructor. Because const widgets require all parameters to be constants, introducing a variable disrupts this and causes an error.
2.2 Common Scenarios Leading to This Error
One common source of the Flutter Invalid Constant Value Using Variable as Parameter error is using runtime data within a const widget. For example, dynamically calculated values, variables, or user inputs will lead to this error when placed within a const constructor.
3. Example of the Error
Let’s illustrate the Flutter Invalid Constant Value Using Variable as Parameter error with a code example:
final int itemCount = 5;
class MyWidget extends StatelessWidget {
const MyWidget({Key? key, required this.count}) : super(key: key);
final int count;
@override
Widget build(BuildContext context) {
return Container(
child: Text('$count items'),
);
}
}
void main() {
runApp(const MyWidget(count: itemCount)); // Error: Flutter Invalid Constant Value Using Variable as Parameter
}
Output:
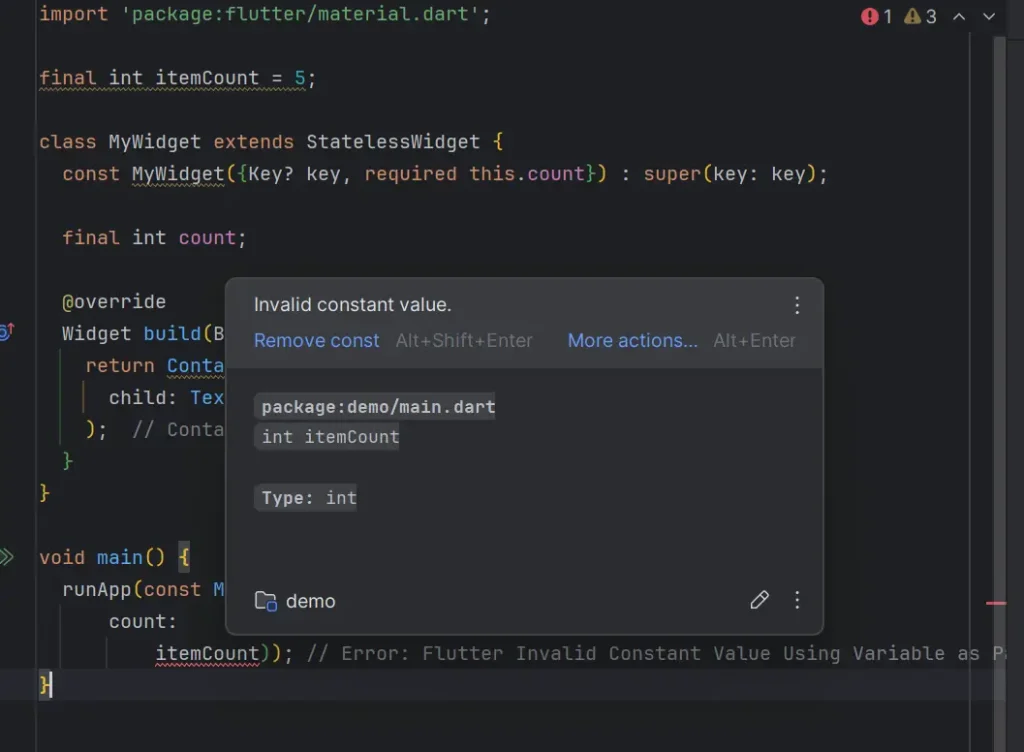
3.1 Why the Error Happens
The code above triggers a Flutter Invalid Constant Value Using Variable as Parameter error because itemCount is a runtime value. Since MyWidget is declared with a const constructor, all parameters must also be constants, which is not the case here.
4. Solutions and Best Practices
To solve the Flutter Invalid Constant Value Using Variable as Parameter error, consider the following approaches:
4.1 Removing the const Keyword
The simplest way to resolve the Flutter Invalid Constant Value Using Variable as Parameter error is to remove const from the widget declaration. By doing so, Flutter allows variable parameters, eliminating the need for compile-time constants.
void main() {
runApp(MyWidget(count: itemCount)); // No error
}
Output:
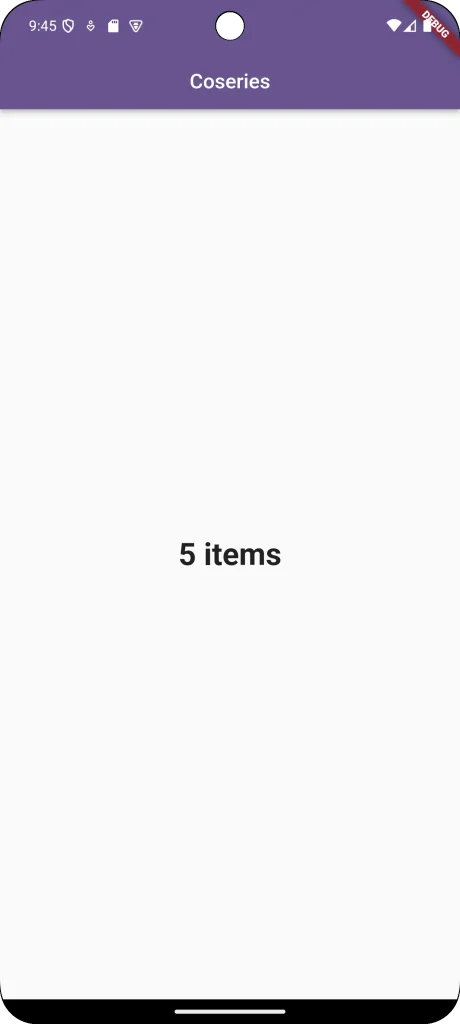
4.2 Using const with Constant Values Only
A good practice to avoid the Flutter Invalid Constant Value Using Variable as Parameter error is to ensure all values passed to const widgets are compile-time constants. This approach optimizes performance while ensuring code stability.
const int itemCount = 5;
void main() {
runApp(const MyWidget(count: itemCount)); // Works as itemCount is const
}
4.3 Using final or var Instead of const
When dealing with values assigned at runtime, use final instead of const. Unlike const, final allows runtime values, sidestepping the Flutter Invalid Constant Value Using Variable as Parameter issue.
4.4 Constructor Parameters
To handle dynamic data in constructors without triggering the Flutter Invalid Constant Value Using Variable as Parameter error, remove the const requirement from the widget’s declaration. This approach ensures that your widget accepts variable values as needed.
5. Advanced Tips and Common Pitfalls
5.1 Stateless vs. Stateful Widgets
When dealing with constants, stateless widgets work best because they don’t change once built. Stateful widgets, on the other hand, can handle runtime values without causing the Flutter Invalid Constant Value Using Variable as Parameter error, so use them when your UI requires dynamic data.
5.2 Performance Considerations
Using const widgets can significantly improve performance. However, to prevent the Flutter Invalid Constant Value Using Variable as Parameter error, use const only with compile-time constants, and avoid forcing constant usage where it’s not suitable.
5.3 Nested Constants
In cases where you have deeply nested constant structures, ensure each level adheres to constant rules. If any layer is non-constant, the Flutter Invalid Constant Value Using Variable as Parameter error may occur.
6. Conclusion
In summary, the Flutter Invalid Constant Value Using Variable as Parameter error is a common but avoidable issue in Flutter. By understanding how const works and ensuring only constant values are used where required, you can write more efficient, error-free code. Following best practices, such as using const for compile-time values and switching to final or var for runtime values, helps maintain flexibility while optimizing app performance. Avoiding the Flutter Invalid Constant Value Using Variable as Parameter error can lead to a smoother development experience and a more efficient Flutter app.
7. Additional Resources
Links
You can read also: How to Get Assets Image Path In Flutter