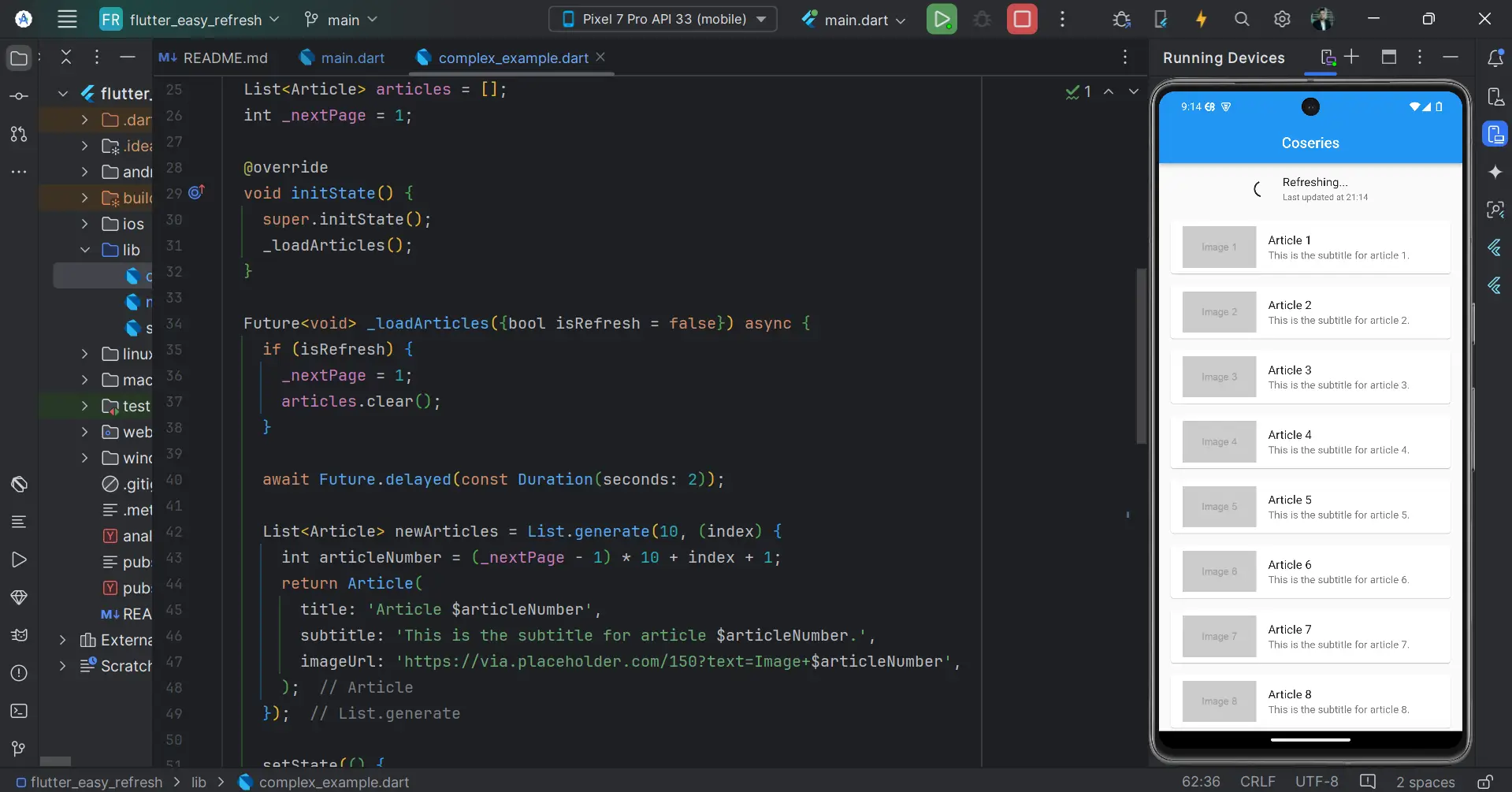
Flutter EasyRefresh: Simplify Your Content Updates Effortlessly
in Flutter on August 28, 2024by Kawser MiahThe Flutter EasyRefresh package is an essential tool for Flutter developers looking to implement smooth and responsive pull-to-refresh and load-more functionalities in their applications. Its versatility and ease of integration make it ideal for apps that require dynamic content loading, such as news feeds, social media timelines, or product catalogs.
Table of Contents
Flutter EasyRefresh
1.1. Overview of Flutter EasyRefresh Package
The Flutter EasyRefresh package for Flutter is designed to provide developers with an intuitive and customizable way to implement pull-to-refresh and load-more functionalities. It is especially beneficial for applications that frequently retrieve data from a server and need to display this data in a list or grid format. By integrating this package, you can enhance the user experience by allowing users to refresh content effortlessly and load more data as they scroll.
1.2. Common Use Cases
Imagine a news app where users can pull down to refresh the latest headlines or scroll down to load more articles. Or think about a social media app where users can pull down to see the newest posts and scroll down to load older ones. The Easy Refresh package excels in these scenarios by offering a seamless and responsive interface that handles these actions smoothly.
2. Key Features of Flutter EasyRefresh
2.1. Customizable Pull-to-Refresh
One of the standout features of the Easy Refresh package is its customizable pull-to-refresh functionality. Developers can easily adjust the appearance and behavior of the refresh indicator to align with their app’s design and UX requirements. Whether you prefer a simple circular progress indicator or a more complex custom animation, Easy Refresh provides the flexibility you need.
2.2. Load-More Functionality
In addition to pull-to-refresh, Easy Refresh also supports load-more functionality, allowing users to retrieve additional data as they reach the end of a list or grid. This is particularly useful for applications that need to handle large datasets by loading them incrementally, thereby improving performance and reducing initial load times.
2.3. Advanced Customization
Beyond the basic pull-to-refresh and load-more features, Easy Refresh offers advanced customization options, such as setting up different refresh and load behaviors, customizing the loading indicators, and even integrating with state management solutions like Riverpod or Provider. This allows developers to create a highly tailored and responsive user experience.
3. Installation and Setup
3.1. Adding the Dependency
To get started with Easy Refresh, add the package to your pubspec.yaml file:
dependencies:
easy_refresh: ^3.4.0 //Replace with the latest version
3.2. Importing the Package
Next, import the Easy Refresh package into your Dart file:
import 'package:easy_refresh/easy_refresh.dart';
4. Basic Usage Example
4.1. Implementing Pull-to-Refresh
The following example demonstrates how to implement a basic pull-to-refresh feature using the Easy Refresh package:
EasyRefresh(
onRefresh: () async {
// Simulate a network request
await Future.delayed(Duration(seconds: 2));
// Update your data source here
},
child: ListView.builder(
itemCount: 20,
itemBuilder: (context, index) {
return ListTile(title: Text('Item $index'));
},
),
);
4.2. Implementing Load-More
Similarly, implementing load-more functionality is straightforward:
EasyRefresh(
onLoad: () async {
// Simulate a network request
await Future.delayed(const Duration (seconds:2));
// Update your data source here
},
child: ListView.builder(
itemCount: 20,
itemBuilder: (context, index) {
return ListTile(
title: Text('Item $index'),
);
},
),
4.3. Example 1
Here is a complete example that combines both pull-to-refresh and load-more functionalities:
import 'package:easy_refresh/easy_refresh.dart';
import 'package:flutter/material.dart';
class SimpleEasyRefresh extends StatefulWidget {
const SimpleEasyRefresh({super.key});
@override
State<SimpleEasyRefresh> createState() => _SimpleEasyRefreshState();
}
class _SimpleEasyRefreshState extends State<SimpleEasyRefresh> {
List<int> _items = List.generate(20, (index) => index);
int _nextItem = 20;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('EasyRefresh Example'),
),
body: EasyRefresh(
onRefresh: () async {
await Future.delayed(const Duration(seconds: 2));
setState(() {
_items = List.generate(20, (index) => index);
_nextItem = 20;
});
},
onLoad: () async {
await Future.delayed(const Duration(seconds: 2));
setState(() {
_items.addAll(List.generate(10, (index) => _nextItem++));
});
},
child: ListView.builder(
itemCount: _items.length,
itemBuilder: (context, index) {
return ListTile(
title: Text('Item ${_items[index]}'),
);
},
),
),
);
}
}
Output:
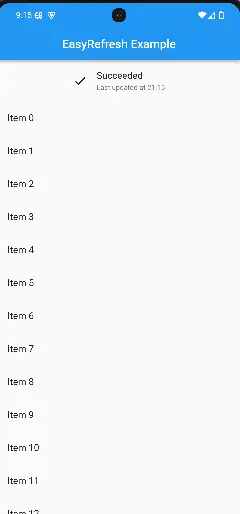
4.4. Example 2
import 'package:flutter/material.dart';
import 'package:easy_refresh/easy_refresh.dart';
class Article {
final String title;
final String subtitle;
final String imageUrl;
Article({
required this.title,
required this.subtitle,
required this.imageUrl,
});
}
class ComplexEasyRefreshExample extends StatefulWidget {
const ComplexEasyRefreshExample({super.key});
@override
State<ComplexEasyRefreshExample> createState() =>
_ComplexEasyRefreshExampleState();
}
class _ComplexEasyRefreshExampleState extends State<ComplexEasyRefreshExample> {
List<Article> articles = [];
int _nextPage = 1;
@override
void initState() {
super.initState();
_loadArticles();
}
Future<void> _loadArticles({bool isRefresh = false}) async {
if (isRefresh) {
_nextPage = 1;
articles.clear();
}
await Future.delayed(const Duration(seconds: 2));
List<Article> newArticles = List.generate(10, (index) {
int articleNumber = (_nextPage - 1) * 10 + index + 1;
return Article(
title: 'Article $articleNumber',
subtitle: 'This is the subtitle for article $articleNumber.',
imageUrl: 'https://via.placeholder.com/150?text=Image+$articleNumber',
);
});
setState(() {
articles.addAll(newArticles);
_nextPage++;
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('EasyRefresh Complex Example'),
),
body: EasyRefresh(
onRefresh: () async {
await _loadArticles(isRefresh: true);
},
onLoad: () async {
await _loadArticles();
},
child: ListView.builder(
itemCount: articles.length,
itemBuilder: (context, index) {
final article = articles[index];
return Card(
margin: const EdgeInsets.symmetric(vertical: 8, horizontal: 16),
child: ListTile(
leading: Image.network(
article.imageUrl,
width: 100,
height: 100,
fit: BoxFit.cover,
),
title: Text(article.title),
subtitle: Text(article.subtitle),
),
);
},
),
),
);
}
}
Output:
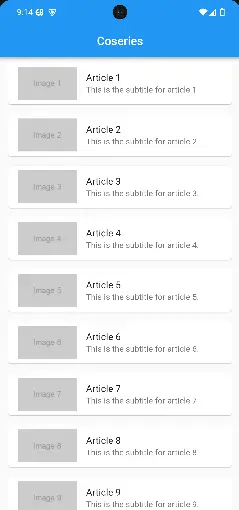
5. Advanced Usage
5.1. Customizing the Refresh Indicator
The Easy Refresh package allows developers to customize the appearance of the refresh indicator. You can change the color, size, and even replace the default indicator with a custom widget to better match your app’s design.
5.2. Integrating with State Management
Integrating Easy Refresh with a state management solution like Riverpod or Provider for more complex applications can help manage the loading state, update the UI efficiently, and handle data more robustly.
5.3. Handling Complex Scenarios
Easy Refresh is versatile enough to handle complex scenarios, such as refreshing multiple lists, paginating through large datasets, or integrating with offline data storage. This makes it a valuable tool for developers working on sophisticated Flutter applications.
6. Full Code
main.dart
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
theme: ThemeData.light(useMaterial3: false),
home: const ComplexEasyRefreshExample(),
);
}
}
class Article {
final String title;
final String subtitle;
final String imageUrl;
Article({
required this.title,
required this.subtitle,
required this.imageUrl,
});
}
class ComplexEasyRefreshExample extends StatefulWidget {
const ComplexEasyRefreshExample({super.key});
@override
State<ComplexEasyRefreshExample> createState() =>
_ComplexEasyRefreshExampleState();
}
class _ComplexEasyRefreshExampleState extends State<ComplexEasyRefreshExample> {
List<Article> articles = [];
int _nextPage = 1;
@override
void initState() {
super.initState();
_loadArticles();
}
Future<void> _loadArticles({bool isRefresh = false}) async {
if (isRefresh) {
_nextPage = 1;
articles.clear();
}
await Future.delayed(const Duration(seconds: 2));
List<Article> newArticles = List.generate(10, (index) {
int articleNumber = (_nextPage - 1) * 10 + index + 1;
return Article(
title: 'Article $articleNumber',
subtitle: 'This is the subtitle for article $articleNumber.',
imageUrl: 'https://via.placeholder.com/150?text=Image+$articleNumber',
);
});
setState(() {
articles.addAll(newArticles);
_nextPage++;
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
centerTitle: true,
title: const Text('Coseries'),
),
body: EasyRefresh(
onRefresh: () async {
await _loadArticles(isRefresh: true);
},
onLoad: () async {
await _loadArticles();
},
child: ListView.builder(
itemCount: articles.length,
itemBuilder: (context, index) {
final article = articles[index];
return Card(
margin: const EdgeInsets.symmetric(vertical: 8, horizontal: 16),
child: ListTile(
leading: Image.network(
article.imageUrl,
width: 100,
height: 100,
fit: BoxFit.cover,
),
title: Text(article.title),
subtitle: Text(article.subtitle),
),
);
},
),
),
);
}
}
Output:
7. Conclusion
7.1. Summary
The Easy Refresh package is a powerful, flexible, and user-friendly tool for adding pull-to-refresh and load-more functionalities to your Flutter applications. Its ease of use, combined with its extensive customization options, makes it a must-have for any Flutter developer looking to enhance the user experience.
7.2. Final Thoughts
By leveraging the Flutter Easy Refresh package, you can significantly improve the interactivity and responsiveness of your app, creating a seamless and enjoyable experience for your users. Whether you’re building a simple app with basic refresh needs or a complex application with advanced data handling, Easy Refresh has you covered. Explore its features, customize it to fit your needs, and take your Flutter app to the next level.
8. Additional Resources
8.1. Links
You can read also: Enhance Your App’s Functionality With Speech to Text