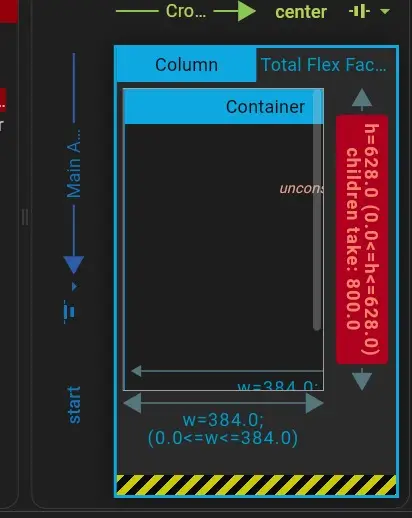
Mastering Flutter Column Overflow: Common Issues and Effective Solutions
in Flutter on September 4, 2024by Kawser Miah
Flutter Column Overflow can be a common issue where widgets within a Column overflow, leading to unexpected layout problems. This is a challenge many developers face. However, by understanding the underlying causes and applying effective solutions, you can simplify your development process. In this article, we will explore the typical scenarios that lead to Flutter Column Overflow, with a particular focus on managing container overflow
Table of Contents
Flutter Column Overflow
Introduction to Flutter Column Widget
Understanding the Flutter Column Widget
The Column widget in Flutter is a core layout component that arranges its child widgets vertically. Unlike the Row widget, which arranges children horizontally, the Column widget stacks them from top to bottom.
Column(
children: <Widget>[
Icon(Icons.star, size: 50),
const Text('Star'),
],
)
In the example above, the Column widget contains an Icon widget and a Text widget, displaying them one below the other. However, as with any layout, improper management of space can lead to Flutter Column Overflow issues.
Key Properties of Column Widget
Column Widget Attributes
The Column widget includes several properties to control the alignment and behavior of its children:
- MainAxisAlignment: Aligns children vertically along the main axis (top to bottom). Options include start, end, center, and space-evenly.
- CrossAxisAlignment: Aligns children horizontally along the cross-axis. Options include start, end, center, and stretch.
- MainAxisSize: Determines whether the Column should occupy the minimum space or expand to fill the available vertical space.
Column(
mainAxisAlignment: MainAxisAlignment.center,
crossAxisAlignment: CrossAxisAlignment.stretch,
children: <Widget>[
Icon(Icons.star, size: 50),
const Text('Star'),
],
)
These properties give you flexibility in managing space, but misconfiguration can lead to Flutter Column Overflow, especially when working with fixed-size widgets like Containers.
Exploring Flutter Column Overflow
What is Flutter Column Overflow?
Flutter Column Overflow occurs when the combined height of the child widgets exceeds the available vertical space within the Column. This often happens when a widget, like a Container with a fixed height, occupies more space than intended, leading to overflow errors.
Column(
children: <Widget>[
Container(
height: 800,
width: 400,
decoration: BoxDecoration(
border: Border.all(),
color: Colors.blue,
),
),
],
),
Visual Indicators of Overflow
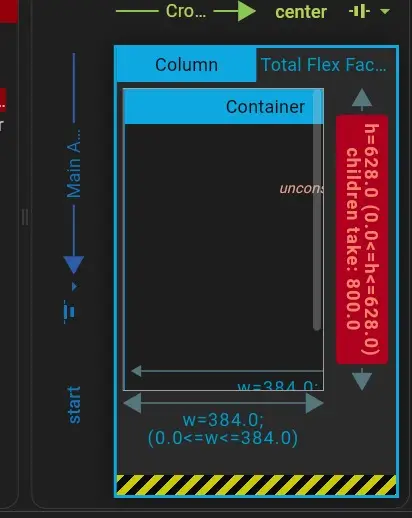
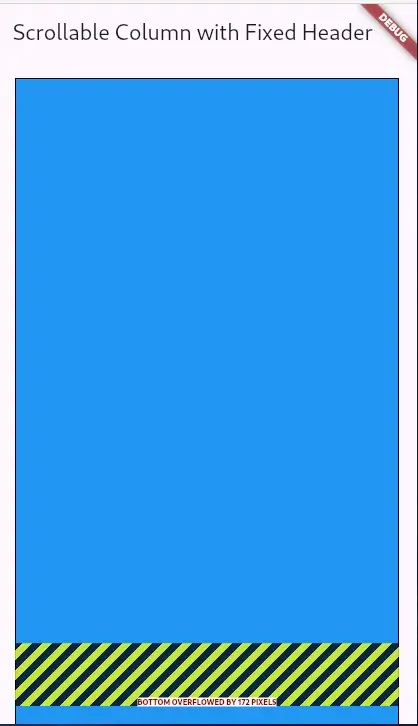
In the example above, the Container widget might occupy more vertical space than available, resulting in Flutter Column Overflow.
Common Scenarios of Flutter Column Overflow
Text Widget in a Column
One common scenario is when a Text widget has too much content to fit within the vertical space of the Column. The text can spill over, causing layout issues.
Column(
children: <Widget>[
const Text('This is an extremely long text that will likely overflow the column'),
Icon(Icons.star, size: 50),
],
)
Image Widget in a Column
Another scenario is using an Image widget with a height that exceeds the available space, causing the image to overflow.
Column(
children: <Widget>[
Image.network('https://example.com/image.jpg', height: 500),
Icon(Icons.star, size: 50),
],
)
Container Overflow in a Column
Container widgets with fixed heights are often the culprits behind Flutter ColumnOverflow. When a Container’s height exceeds the available space, it forces the other widgets to overflow.
Column(
children: <Widget>[
Container(
height: 500,
child: const Text('This container is too tall for the column'),
),
Icon(Icons.star, size: 50),
],
)
In this example, the Container’s height exceeds the available vertical space, pushing the Icon widget out of view, resulting in Flutter Column Overflow.
Detecting and Resolving Flutter Column Overflow
Using the Expanded Widget
One solution to Flutter Column Overflow is the Expanded widget, allowing a child to take up the remaining space in the main axis direction.
Column(
children: <Widget>[
Expanded(
child: Container(
child: const Text('This container will expand to fit the available space'),
),
),
Icon(Icons.star, size: 50),
],
)
Using the Flexible Widget
The Flexible widget gives more control over how much space a child should take relative to other children, helping to manage Flutter Column Overflow.
Column(
children: <Widget>[
Flexible(
flex: 2,
child: Container(
color: Colors.blue,
child: const Text('Flexible widget with flex factor of 2'),
),
),
Flexible(
flex: 1,
child: Icon(Icons.star, size: 50),
),
],
)
Using SingleChildScrollView
To avoid overflow, wrapping the Column in a SingleChildScrollView allows the content to scroll instead of overflowing, effectively preventing Flutter Column Overflow.
SingleChildScrollView(
child: Column(
children: <Widget>[
Container(
height: 500,
child: const Text('This container is too tall for the column but scrollable'),
),
Icon(Icons.star, size: 50),
],
),
)
Best Practices to Prevent Flutter Column Overflow
Utilizing Constraints Properly
Ensure that the combined height of children does not exceed the available space. Applying constraints or using Expanded/Flexible widgets can help manage this effectively, avoiding Flutter Column Overflow.
Designing for Responsiveness
Design your layout to adapt to different screen sizes. Use MediaQuery or LayoutBuilder to create responsive designs that avoid Flutter Column Overflow on smaller screens.
Regular Debugging and Testing
Test your app on various devices and screen orientations to catch and fix overflow issues early. Regular debugging is key to maintaining a stable UI and preventing Flutter ColumnOverflow.
Overflow-Free Column Management
Dealing with Flutter Column Overflow can be challenging, but it’s manageable with the right approach and tools. Understanding the causes and applying solutions like Expanded, Flexible, and SingleChildScrollView can help you prevent and resolve overflow issues.
Proper use of constraints and responsive design, coupled with regular testing, will ensure your Flutter apps provide a smooth user experience across all devices.
Handle Flutter ColumnOverflow like a pro! Build dynamic, responsive Flutter UIs effortlessly with DhiWise Flutter Builder. Create beautiful, overflow-free layouts with pre-built widgets, visual editing, and real-time previews. Try it and make your UI development process smoother and more efficient!
You can read also: Create Stunning Flutter Calendar UIs with Calendar Carousel