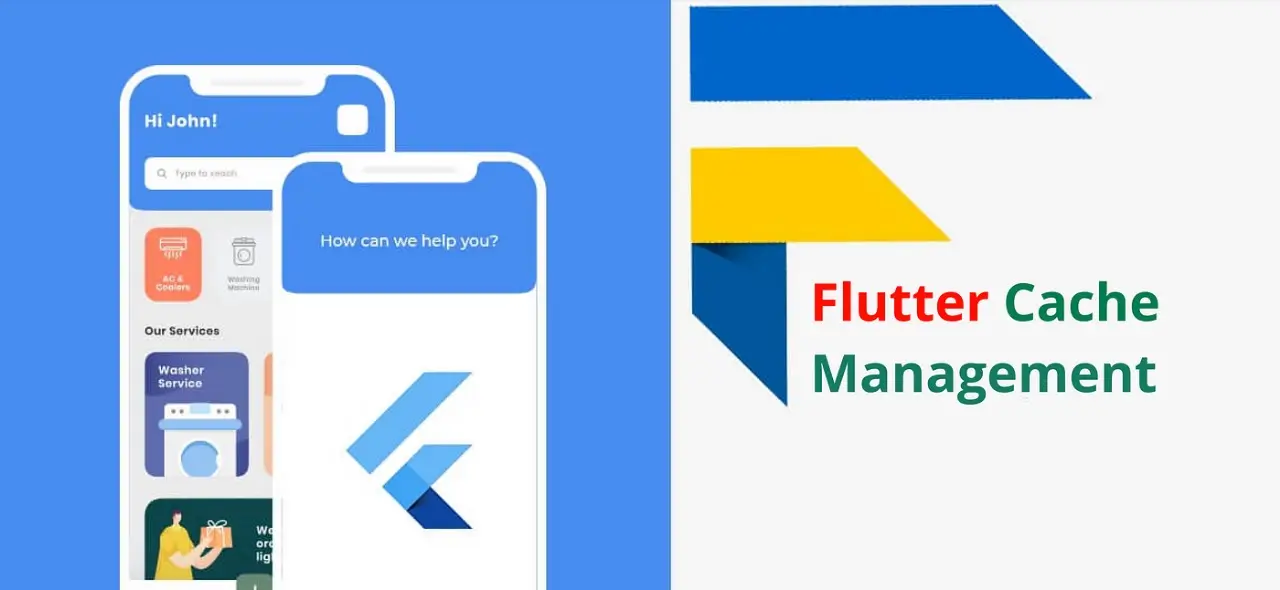
Flutter CacheManager: Efficient data caching for your flutter Application
in Flutter on August 22, 2024by Kawser MiahThe Flutter CacheManager package is an essential tool for developers looking to implement efficient and reliable caching in their Flutter applications. By caching data such as images, files, or any other network resource, this package significantly improves app performance and user experience. It is particularly useful for apps that require offline support or need to minimize network requests.
Table of Contents
Flutter CacheManager
1.1 Brief Overview of Flutter Cache Manager
The Flutter Cache Manager is designed to simplify caching within your Flutter app. It handles the downloading and caching of files in a way that is both easy to implement and powerful enough to meet various caching needs. Whether you are working on a media-rich application or a data-heavy app, this package can help reduce load times and optimize network usage.
1.2 Context
Imagine developing a news app where images need to be downloaded and displayed for various articles. With the Flutter CacheManager, these images can be cached locally, ensuring they load instantly when the user revisits an article. Similarly, if you are building a weather app, caching weather icons or other resources can significantly enhance the user experience, especially in areas with poor internet connectivity.
2.Key Features
The Flutter CacheManager package offers several key features that make it an indispensable tool for Flutter developers:
2.1 Easy Integration
Integrating the cache manager into your app is straightforward. The package provides a simple API to download and cache files, allowing you to focus more on your app’s core functionality rather than the intricacies of caching.
2.2 Customizable Cache Configuration
The package allows you to configure various aspects of the cache, such as the maximum cache size, file age, and custom file service. This flexibility ensures that you can tailor the caching behavior to suit your app’s specific requirements.
2.3 Automatic Cache Management
The Flutter CacheManager handles cache expiration and removal automatically. This ensures that your app does not consume excessive storage space while still maintaining quick access to frequently used resources.
3. Installation and Setup
3.1 Adding the Dependency
To get started, add the Flutter CacheManager package to your pubspec.yaml file:
dependencies:
flutter_cache_manager: ^3.4.1 //Replace with the latest version
path_provider: ^2.1.4 //Replace with the latest version
3.2 Importing the Package
After adding the dependency, import the package into your Dart file:
import 'package:flutter_cache_manager/flutter_cache_manager.dart';
4. Basic Usage
Here’s an example of how to use the Flutter CacheManager to download and cache an image:
FutureBuilder<File>(
future: DefaultCacheManager().getSingleFile(url),
builder: (context, snapshot) {
if (snapshot.connectionState == ConnectionState.done &&
snapshot.hasData) {
return Padding(
padding: const EdgeInsets.all(18.0),
child: Column(
children: [
const Text(
'Example Picture Using Flutter cache: ',
style: TextStyle(
fontSize: 20,
fontWeight: FontWeight.bold,
),
),
const SizedBox(
height: 50,
),
Image.file(
snapshot.data!,
),
],
),
);
} else if (snapshot.hasError) {
return const Center(
child: Text('Error loading image'),
);
}
return const Center(
child: CircularProgressIndicator(),
);
},
),
OutPut:
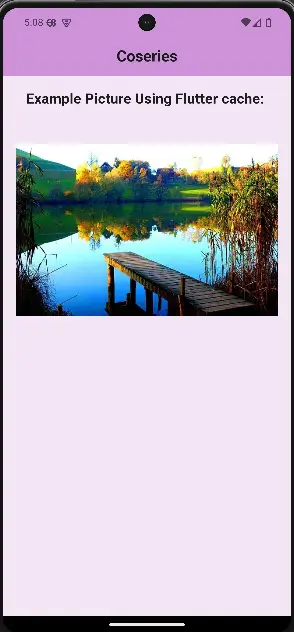
5. Advanced Usage
5.1.Customized Cache Manager
The cache manager is customizable by creating a new CacheManager. It is very important to not create more than 1 CacheManager instance with the same key as these bite each other. In the example down here the manager is created as a Singleton, but you could also use for example Provider to Provide a CacheManager on the top level of your app. Below is an example with other settings for the maximum age of files, maximum number of objects and a custom FileService. The key parameter in the constructor is mandatory, all other variables are optional.
import 'package:flutter_cache_manager/flutter_cache_manager.dart';
class CustomCacheManager {
static const key = 'customCacheKey';
static CacheManager instance = CacheManager(
Config(
key,
stalePeriod: const Duration(days: 7),
maxNrOfCacheObjects: 20,
repo: JsonCacheInfoRepository(databaseName: key),
fileSystem: IOFileSystem(key),
fileService: HttpFileService(),
),
);
}
5.2.Clearing the Cache
You might want to clear the cache manually under certain conditions, such as when the user logs out or when the cache exceeds a certain size.
Example: Clearing the Entire Cache
await DefaultCacheManager().emptyCache();
Example: Removing Specific Files from the Cache
final customCacheManager = CustomCacheManager();
await customCacheManager.removeFile('https://example.com/image.jpg');
5.3. Storing Files with a Custom Key
By default, files are cached using their URL as the key. However, you might want to store files with a custom key, which can be useful for accessing the same file through different URLs.
Example: Custom Key for Caching
final customCacheManager = CustomCacheManager();
await customCacheManager.putFile(
'custom_key',
file.readAsBytesSync(), // File content as bytes
eTag: 'my_unique_identifier', // Optional identifier for the file version
);
final file = await customCacheManager.getSingleFile('custom_key');
6. Final Code
main.dart
import 'package:flutter/material.dart';
import 'package:flutter_cache_manager/flutter_cache_manager.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
debugShowCheckedModeBanner: false,
home: HomePage(),
);
}
}
class HomePage extends StatefulWidget {
const HomePage({super.key});
@override
State<HomePage> createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
@override
Widget build(BuildContext context) {
String url =
'https://i.pinimg.com/736x/c3/d3/15/c3d315f7216dcf8b320e8825a9f5d126.jpg';
return Scaffold(
backgroundColor: Colors.purple[50],
appBar: AppBar(
backgroundColor: Colors.purple[200],
centerTitle: true,
title: const Text(
'Coseries',
style: TextStyle(
fontWeight: FontWeight.bold,
),
),
),
body: FutureBuilder<File>(
future: DefaultCacheManager().getSingleFile(url),
builder: (context, snapshot) {
if (snapshot.connectionState == ConnectionState.done &&
snapshot.hasData) {
return Padding(
padding: const EdgeInsets.all(18.0),
child: Column(
children: [
const Text(
'Example Picture Using Flutter cache: ',
style: TextStyle(
fontSize: 20,
fontWeight: FontWeight.bold,
),
),
const SizedBox(
height: 50,
),
Image.file(
snapshot.data!,
),
],
),
);
} else if (snapshot.hasError) {
return const Center(
child: Text('Error loading image'),
);
}
return const Center(
child: CircularProgressIndicator(),
);
},
),
);
}
}
7. Conclusion
7.1. Summary
The Flutter CacheManager package is a robust solution for implementing caching in Flutter applications. Its ease of use, customizable configuration, and automatic management make it a valuable tool for developers.
7.2.Final Thoughts
By leveraging the Flutter CacheManager, you can significantly improve your app’s performance and user experience. Whether you need to cache images, files, or other network resources, this package offers the tools you need to implement efficient caching with minimal effort.
8. Additional Resources
8.1.Links
You can read also: Efficient and Reliable Scanning Solution