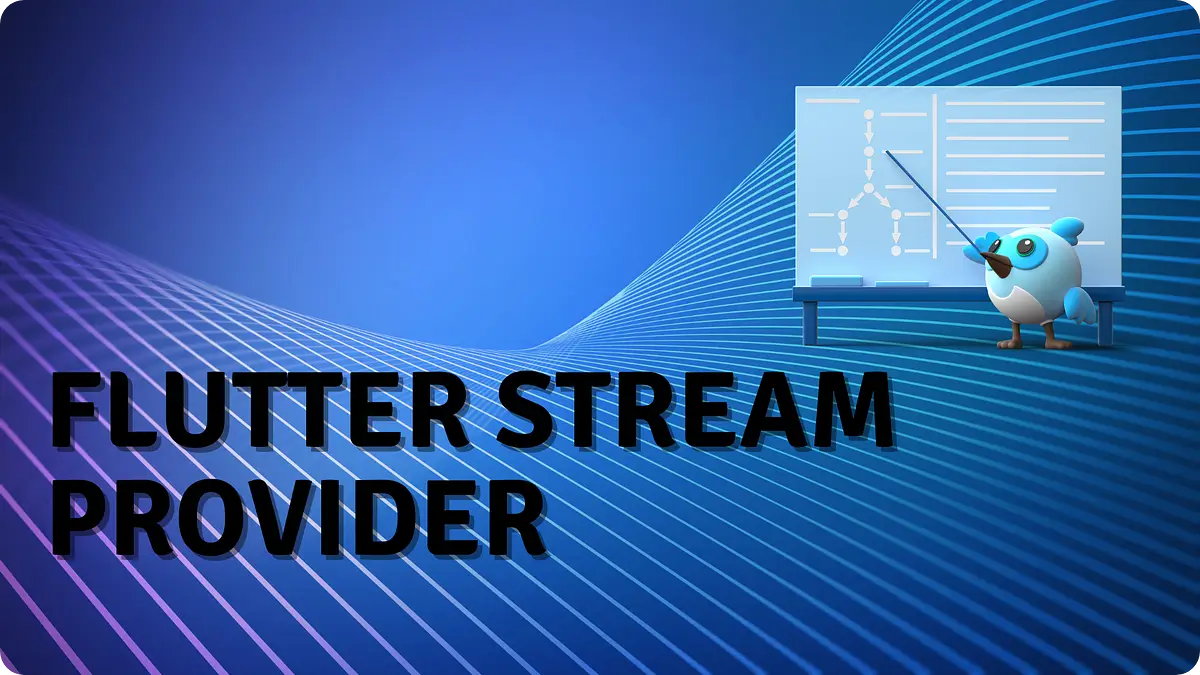
Stream in Provider with Flutter: Enhance Your App’s Performance
in Flutter on December 17, 2024by Kawser MiahStream in Provider with Flutter is a powerful combination for managing asynchronous data and real-time updates in Flutter applications. It allows you to build responsive and high-performing apps by seamlessly integrating Streams with the Provider package. In this guide, we’ll explore how to use Stream in Provider with Flutter to handle asynchronous data effectively, ensuring your app stays responsive and delivers a smooth user experience.
Table of Contents
Stream in Provider with Flutter
What is a Stream in Flutter?
A Stream in Flutter is an asynchronous sequence of data events. It allows you to handle data updates over time, such as user inputs, network responses, or real-time notifications. Using a stream in Provider with Flutter lets you listen to these updates efficiently and update the UI automatically.
Why Combine Stream with Provider?
Integrating a stream in Provider with Flutter is a great choice for several reasons:
- Real-Time Updates: A stream in Provider with Flutter enables real-time UI updates when the underlying data changes.
- Simplified State Management: Provider simplifies dependency injection, making it easier to use streams in your app.
- Improved App Performance: Using a stream in Provider with Flutter minimizes unnecessary widget rebuilds, focusing updates only where needed.
Step-by-Step Guide to Using Stream in Provider with Flutter:
Here’s a detailed walkthrough to integrate a stream in Provider with Flutter effectively.
Step 1: Add Dependencies
First, add the provider package to your pubspec.yaml file:
dependencies:
flutter:
sdk: flutter
provider: ^6.1.2
Run flutter pub get to fetch the package.
Step 2: Create a Stream
Next, let’s create a simple stream that emits data. For example, let’s create a stream that emits the current time every second:
Stream<String> timeStream() async* {
while (true) {
await Future.delayed(Duration(seconds: 1));
yield DateTime.now().toString();
}
}
This stream will emit the current date and time every second.
Step 3: Provide the Stream
To provide the stream to the widget tree, use StreamProvider. You can wrap your root widget or any specific widget where you want to access the stream.
void main() {
runApp(
MultiProvider(
providers: [
StreamProvider<String>(create: (_) => timeStream(), initialData: 'Loading...'),
],
child: MyApp(),
),
);
}
Step 4: Consume the Stream in Widgets
To consume the stream data in your widgets, use Consumer or context.watch with the stream’s provided data. Here’s an example of how to display the current time:
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text("Stream in Provider Example")),
body: Center(
child: Consumer<String>(
builder: (context, time, child) {
return Text(
'Current time: $time',
style: TextStyle(fontSize: 24),
);
},
),
),
),
);
}
}
In this example, the Consumer widget listens to changes in the stream provided by the StreamProvider. Whenever the stream emits new data, the Consumer rebuilds the widget to reflect the new time.
Handling Errors in Streams
Handling errors in streams is important for robust app behavior. You can use the StreamProvider’s error handling capabilities to deal with errors gracefully.
For example, to handle errors in the stream:
Stream<int> numberStream() async* {
try {
yield* Stream.periodic(Duration(seconds: 1), (count) => count).take(10);
} catch (e) {
throw 'Stream error: $e';
}
}
To display errors in your widget, you can modify the Consumer widget to listen for stream errors:
Consumer<int>(
builder: (context, count, child) {
if (count == null) {
return CircularProgressIndicator();
} else if (count == -1) {
return Text('Error occurred');
}
return Text('Count: $count');
},
)
Combining Streams in Provider
One powerful feature of Stream in Provider with Flutter is the ability to combine multiple streams into one. You can use StreamZip or StreamGroup to combine multiple streams and emit data when all the streams have new values. Here’s an example of combining two streams:
Stream<int> streamOne() async* {
await Future.delayed(Duration(seconds: 1));
yield 1;
}
Stream<int> streamTwo() async* {
await Future.delayed(Duration(seconds: 2));
yield 2;
}
Stream<List<int>> combinedStream() {
return StreamGroup.merge([streamOne(), streamTwo()]);
}
In this example, both streams will emit their values, and the combined stream will emit a list containing values from both.
Example code:
import 'package:flutter/material.dart';
import 'package:provider/provider.dart';
/// A stream that emits the current date and time every second
Stream<String> timeStream() async* {
while (true) {
await Future.delayed(const Duration(seconds: 1));
yield DateTime.now().toString();
}
}
void main() {
runApp(
MultiProvider(
providers: [
// Providing the timeStream as a StreamProvider
StreamProvider<String>(
create: (_) => timeStream(),
initialData: 'Loading...',
),
],
child: const MyApp(),
),
);
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Stream in Provider Example',
debugShowCheckedModeBanner: false,
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: HomePage(),
);
}
}
class HomePage extends StatelessWidget {
const HomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text(
'Stream in Provider Example',
style: TextStyle(fontWeight: FontWeight.bold),
),
centerTitle: true,
),
body: Container(
decoration: const BoxDecoration(
gradient: LinearGradient(
colors: [Colors.blueAccent, Colors.lightBlue, Colors.white],
begin: Alignment.topCenter,
end: Alignment.bottomCenter,
),
),
child: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Icon(
Icons.access_time_rounded,
size: 100,
color: Colors.blue.shade700,
),
const SizedBox(height: 20),
Consumer<String>(
builder: (context, time, child) {
return Text(
time,
style: TextStyle(
fontSize: 28,
fontWeight: FontWeight.bold,
color: Colors.blue.shade900,
),
);
},
),
],
),
),
),
);
}
}
Output:
Benefits of Using Stream in Provider with Flutter
Here’s why you should use stream in Provider with Flutter:
- Real-Time Data Processing: Perfect for apps requiring live updates.
- Simpler State Management: Simplifies your codebase by managing streams efficiently.
- Enhanced Performance: Optimizes UI rebuilds for better responsiveness.
Conclusion
Incorporating streams in Provider with Flutter is a powerful way to manage asynchronous data and state changes in your Flutter applications. Whether you’re handling real-time data, updating the UI in response to network calls, or managing user inputs, streams in Provider can help make your app more efficient, scalable, and responsive.
Usin StreamProvider and Consumer, Flutter developers can easily create reactive applications that perform well even when dealing with complex state and asynchronous data. Combining streams in Provider also allows for cleaner, more maintainable code by centralizing state management and avoiding the need for complex logic in the UI layer.
So, next time you’re building a Flutter app that needs to handle asynchronous data, make sure to leverage the power of streams in Provider to enhance your app’s performance and responsiveness.
Additional Resources
Links
You can read also: Compare Two TimeOfDay Instances The Ultimate Guide In Flutter