Flutter How to Compare Two TimeOfDay Instances is an essential task for many features in mobile applications, such as scheduling, reminders, and time-based validations. The TimeofDay class in Flutter provides a simple way to handle time efficiently. A common requirement is comparing two TimeofDay objects to determine whether one time is earlier, later, or equal to another. This comprehensive guide will walk you through the best practices and examples for comparing TimeofDay instances in Flutter.
Table of Contents
Flutter How to Compare Two TimeOfDay Instances
What is TimeOfDay in Flutter?
The TimeOfDay class represents a time of day in hours and minutes, typically in a 24-hour format. It’s a lightweight solution for time handling, especially when dates are not involved. However, it lacks direct comparison methods, requiring developers to implement their own logic.
Why Compare Two TimeOfDay Times?
Comparing two TimeOfDay times is a common use case in many scenarios:
- Validating Input: Ensuring a selected end time is after the start time.
- Triggering Events: Checking if the current time falls within a range.
- Scheduling Tasks: Determining order or precedence of tasks.
Methods to Compare Two TimeOfDay Times
Here are some proven techniques to handle comparisons between two TimeOfDay instances.
1. Converting TimeOfDay to Total Minutes
A practical approach is converting each TimeOfDay to the total minutes since midnight. This makes comparison straightforward.
bool isBefore(TimeOfDay time1, TimeOfDay time2) {
 int minutes1 = time1.hour * 60 + time1.minute;
 int minutes2 = time2.hour * 60 + time2.minute;
 return minutes1 < minutes2;
}
bool isAfter(TimeOfDay time1, TimeOfDay time2) {
 int minutes1 = time1.hour * 60 + time1.minute;
 int minutes2 = time2.hour * 60 + time2.minute;
 return minutes1 > minutes2;
}
bool isEqual(TimeOfDay time1, TimeOfDay time2) {
 return time1.hour == time2.hour && time1.minute == time2.minute;
}//Flutter How to Compare Two TimeOfDay Instances
Using these functions, you can determine if one time is before, after, or equal to another.
2. Leveraging DateTime for Comparisons
Another effective way to compare two TimeOfDay times in Flutter is by converting them to DateTime objects. This method utilizes Flutter’s built-in DateTime comparison functions like isBefore and isAfter.
DateTime convertToDateTime(TimeOfDay time) {
 final now = DateTime.now();
 return DateTime(now.year, now.month, now.day, time.hour, time.minute);
}
bool isBefore(TimeOfDay time1, TimeOfDay time2) {
 return convertToDateTime(time1).isBefore(convertToDateTime(time2));
}
bool isAfter(TimeOfDay time1, TimeOfDay time2) {
 return convertToDateTime(time1).isAfter(convertToDateTime(time2));
}//Flutter How to Compare Two TimeOfDay Instances
This method is especially useful when you need to integrate time comparisons with dates.
Practical Example
void main() {
 TimeOfDay start = TimeOfDay(hour: 9, minute: 30);
 TimeOfDay end = TimeOfDay(hour: 17, minute: 0);
 if (isBefore(start, end)) {
   print("Start time is before the end time.");
 } else if (isAfter(start, end)) {
   print("Start time is after the end time.");
 } else {
   print("Start time and end time are the same.");
 }
}//Flutter How to Compare Two TimeOfDay Instances
Final Code & Output:
main.dart
import 'package:flutter/material.dart';
void main() => runApp(const MyApp());
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
debugShowCheckedModeBanner: false,
home: HomeScreen(),
);
}
}
class HomeScreen extends StatefulWidget {
const HomeScreen({super.key});
@override
State createState() => _HomeScreenState();
}
class _HomeScreenState extends State<HomeScreen> {
TimeOfDay? _meeting1Start;
TimeOfDay? _meeting1End;
TimeOfDay? _meeting2Start;
TimeOfDay? _meeting2End;
String _resultMessage = '';
/// Method to check for conflicts
void _checkConflict() {
if (_meeting1Start == null ||
_meeting1End == null ||
_meeting2Start == null ||
_meeting2End == null) {
setState(() {
_resultMessage = 'Please select all times.';
});
return;
}
// Convert TimeOfDay to DateTime
final now = DateTime.now();
final meeting1Start = DateTime(
now.year,
now.month,
now.day,
_meeting1Start!.hour,
_meeting1Start!.minute,
);
final meeting1End = DateTime(
now.year,
now.month,
now.day,
_meeting1End!.hour,
_meeting1End!.minute,
);
final meeting2Start = DateTime(
now.year,
now.month,
now.day,
_meeting2Start!.hour,
_meeting2Start!.minute,
);
final meeting2End = DateTime(
now.year,
now.month,
now.day,
_meeting2End!.hour,
_meeting2End!.minute,
);
// Adjust for overnight meetings
final adjustedMeeting2End = meeting2End.isBefore(meeting2Start)
? meeting2End.add(const Duration(days: 1))
: meeting2End;
// Check for conflicts
bool conflict = meeting1Start.isBefore(adjustedMeeting2End) &&
meeting1End.isAfter(meeting2Start);
setState(() {
_resultMessage =
conflict ? 'Meetings conflict!' : 'No conflict, you are good to go!';
});
}
/// Method to pick time
Future<TimeOfDay?> _pickTime(BuildContext context) async {
return await showTimePicker(context: context, initialTime: TimeOfDay.now());
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
centerTitle: true,
backgroundColor: Colors.red[100],
title: const Text('Coseries Meeting Scheduler'),
),
body: Padding(
padding: const EdgeInsets.all(16.0),
child: Column(
children: [
const Text(
'Set Meeting 1 Time:',
style: TextStyle(
fontWeight: FontWeight.bold,
fontSize: 20,
),
),
Row(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: [
TextButton(
onPressed: () async {
_meeting1Start = await _pickTime(context);
setState(
() {},
);
},
child: Text(
style: const TextStyle(fontSize: 20),
'Start: ${_meeting1Start?.format(context) ?? 'Select'}',
),
),
TextButton(
onPressed: () async {
_meeting1End = await _pickTime(context);
setState(
() {},
);
},
child: Text(
style: const TextStyle(fontSize: 20),
'End: ${_meeting1End?.format(context) ?? 'Select'}',
),
),
],
),
const SizedBox(height: 20),
const Text(
'Set Meeting 2 Time:',
style: TextStyle(
fontWeight: FontWeight.bold,
fontSize: 20,
),
),
Row(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: [
TextButton(
onPressed: () async {
_meeting2Start = await _pickTime(context);
setState(() {});
},
child: Text(
style: const TextStyle(fontSize: 20),
'Start: ${_meeting2Start?.format(context) ?? 'Select'}',
),
),
TextButton(
onPressed: () async {
_meeting2End = await _pickTime(context);
setState(() {});
},
child: Text(
style: const TextStyle(fontSize: 20),
'End: ${_meeting2End?.format(context) ?? 'Select'}',
),
),
],
),
const SizedBox(height: 20),
ElevatedButton(
onPressed: _checkConflict,
child: const Text(
'Check Conflict',
style: TextStyle(fontSize: 18),
),
),
const SizedBox(height: 20),
Text(
_resultMessage,
style: TextStyle(
fontSize: 18,
color: _resultMessage.contains('No conflict')
? Colors.green
: Colors.red,
),
),
],
),
),
);
}
}//Flutter How to Compare Two TimeOfDay Instances
Output:
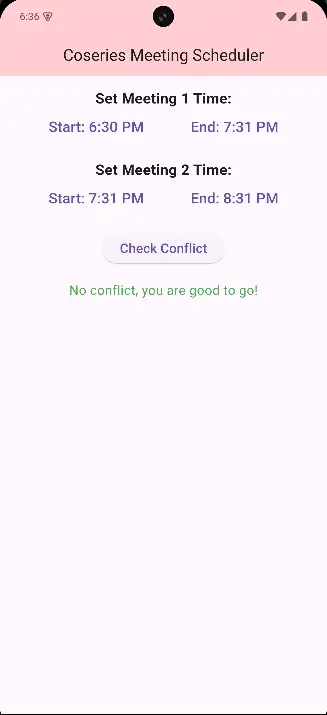
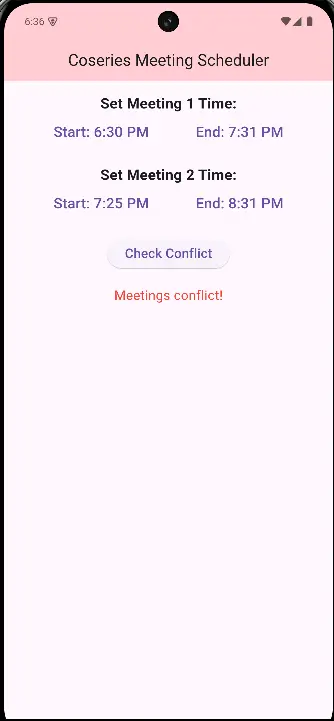
Handling Edge Cases:
When comparing two TimeOfDay times in Flutter, it’s important to consider edge cases:
- Midnight Transition: Ensure your logic accounts for times past midnight.
- User Input Errors: Handle cases where the user selects invalid time ranges.
- Time Zones: If dealing with different time zones, consider using DateTime for accuracy.
Advanced Comparison Scenarios
Beyond simple comparisons, here are a few advanced scenarios:
Time Range Checks: Verify if a specific time falls within a range.
bool isInRange(TimeOfDay target, TimeOfDay start, TimeOfDay end) {
 return isAfter(target, start) && isBefore(target, end);
}//Flutter How to Compare Two TimeOfDay Instances
Sorting Times: Sort a list of TimeOfDay objects using custom comparison logic.
Use Cases for Comparing TimeOfDay Times
Here are some common use cases where you might need to compare two TimeOfDay times in Flutter:
- Reminders: Checking if a scheduled reminder time has passed.
- Meetings: Validating meeting start and end times.
- Alarms: Ensuring alarm times are set correctly relative to the current time.
Best Practices
- Consistent Conversions: Use a single method for all comparisons in your app to maintain consistency.
- Error Handling: Always validate input before performing comparisons.
- Unit Testing: Test your time comparison functions to ensure accuracy.
Conclusion
Mastering how to compare two TimeOfDay times in Flutter is essential for building reliable time-based features in your applications. By using techniques like minute conversion or DateTime conversion, you can perform precise and efficient comparisons. These methods ensure your app handles time comparisons seamlessly, enhancing both functionality and user experience.
Start implementing these strategies today, and elevate the time-handling capabilities of your Flutter apps!
Real-World Example: Comparing Two TimeOfDay Times
You can read also: Invalid Constant Value Using Variable as Parameter In Flutter