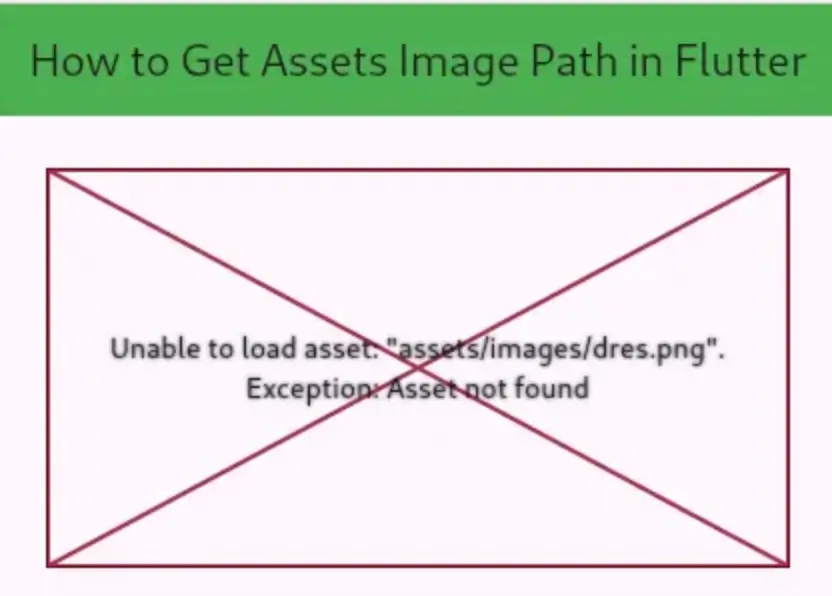
Flutter Asset Not Working is a common problem faced by developers when building mobile apps using the Flutter framework. Sometimes assets like images, fonts, and files do not work as expected, causing frustration. In this guide, we’ll walk through the common causes of asset issues in Flutter and provide quick fixes with code examples and proper project structure. The focus will be on resolving the Flutter Asset Not Working problem, helping you get your project back on track.
Table of Contents
Flutter Asset Not Working
What Are Assets in Flutter?
Assets are external files, such as images, fonts, or data files, bundled with your app during the build process. Flutter’s pubspec.yaml
file allows you to define and include these assets in your project.
Common Causes of Flutter Asset Not Working
1.Incorrect Asset Declaration in pubspec.yaml
The most common cause is incorrectly declaring assets in the pubspec.yaml
file. Even a minor indentation error or missing declaration can prevent assets from loading, resulting in the Flutter Asset Not Working issue.
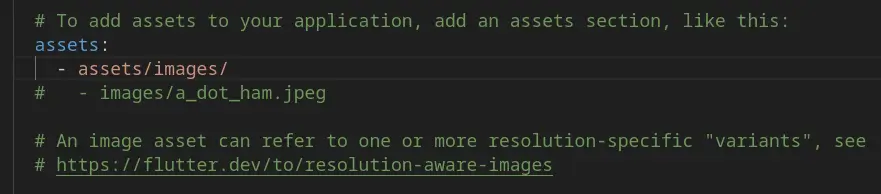
2. Missing or Incorrect Asset Path
If the path to the asset is incorrect or the asset file is missing, Flutter won’t be able to load it.
Container(
height: 200,
width: 180,
decoration: BoxDecoration(
border: Border.all(),
),
child: Image.asset(
'assets/laptop.pn',
height: 180,
fit: BoxFit.fitHeight,
),
),
Output:
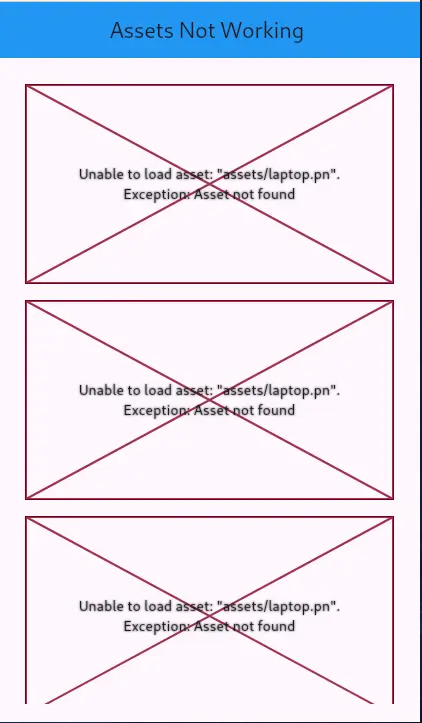
3. Caching Issues During Development
Sometimes, Flutter’s hot-reload or cache may cause the assets not to update properly, even if they are correctly set up.
4. Build Errors Not Related to Assets
Occasionally, build errors unrelated to assets might cause the app to fail to load them properly. This can often be mistaken for an asset issue.
Quick Fixes and Solutions for Flutter Assets Not Working
1. Correctly Declaring Assets in pubspec.yaml
The most important step is to declare your assets properly in the `pubspec.yaml` file. This file manages Flutter dependencies, including your assets.
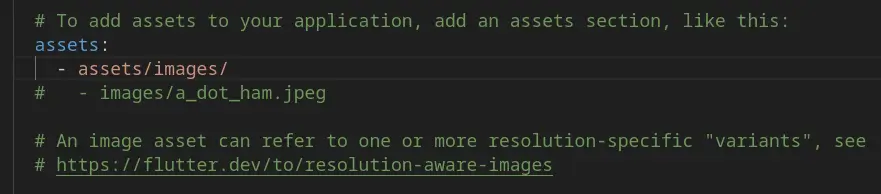
Make sure:
– The file paths are correct.
– The indentation is two spaces.
– The assets folder and files actually exist in the project.
Folder Structure:
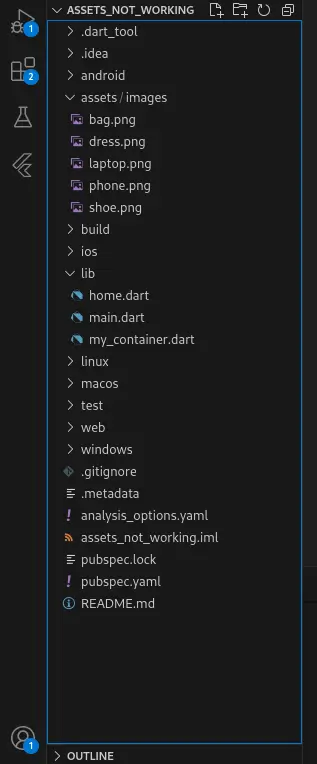
2. Double-Check Asset Paths
Ensure that the path you’ve specified in the `pubspec.yaml` file matches the actual location of the asset in your project. Remember that asset paths are case-sensitive in Flutter. If you have an image laptop.png inside assets/images/, make sure the path is exactly as declared.
3. Force Rebuild and Clear Cache
Sometimes, the problem lies in cached files during development. If you’ve made changes and they are not reflected, you may need to force Flutter to rebuild your app.
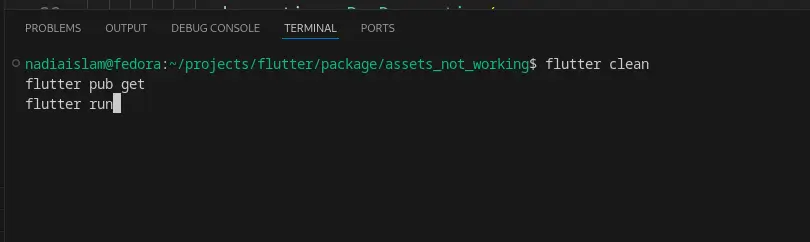
This clears any cached data and forces a fresh build, which often resolves asset issues.
4. Use the Correct Path in Code
When using the Image.asset() or similar methods, ensure that the asset path matches the one declared in the pubspec.yaml.
Example Code:
import 'package:flutter/material.dart';
class MyContainer extends StatelessWidget {
const MyContainer({super.key});
@override
Widget build(BuildContext context) {
return Padding(
padding: const EdgeInsets.all(8.0),
child: Container(
height: 200,
width: 400,
decoration: BoxDecoration(
border: Border.all(),
),
child: Image.asset(
'assets/images/laptop.png',
height: 180,
fit: BoxFit.fitHeight,
),
),
);
}
}
OutPut:
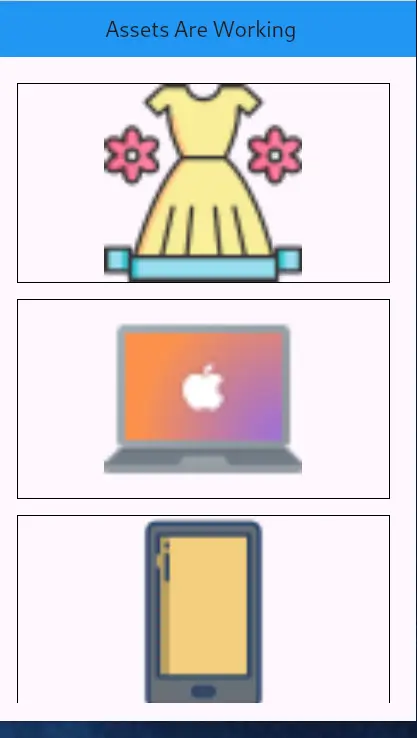
5. Check Asset File Extensions
Ensure that the file extensions (e.g., `.png`, `.jpg`) are correct. Incorrect extensions can also prevent assets from loading properly.
Advanced Troubleshooting
1. Log and Debug Asset Errors
To get detailed insight into asset loading errors, you can inspect the logs during the build and run process. Use the `flutter run` command and watch the terminal output for any asset-related warnings or errors.
2. Use the AssetImage Class
If you want to check whether an image asset is being loaded correctly, you can use the `AssetImage` class to load the image and catch any potential errors.
@override
Widget build(BuildContext context) {
return Image(
image: AssetImage('assets/images/laptop.png'),
);
}
This can help in pinpointing whether the issue lies in how the image is being loaded.
3. Checking Flutter Doctor for Build Issues
Running `flutter doctor` can help you detect any issues with your Flutter installation that might be causing assets not to load properly.
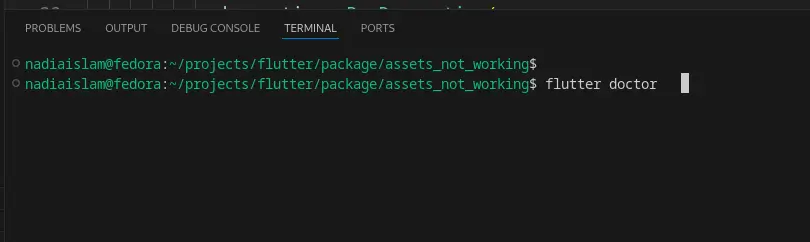
4. Ensure Image Dimensions Are Supported
Sometimes the image file might be corrupted or in a format that Flutter cannot render. Ensure that the image files you use are supported formats such as .png or .jpg and that they have proper dimensions to avoid the Flutter Asset Not Working issue.
Best Practices to Avoid Asset Issues
- Always double-check paths: Ensure all asset paths in
pubspec.yaml
match your folder structure exactly. - Organize assets into folders: It’s a good practice to keep images, fonts, and other assets in clearly named folders (e.g., assets/images, assets/fonts).
- Use relative paths: Ensure paths are relative to the root directory of your Flutter project.
- Keep your
pubspec.yaml
clean: A clutteredpubspec.yaml
file can easily cause errors. Keep it tidy and properly indented to avoid any Flutter Asset Not Working problems.
Conclusion
The Flutter Asset Not Working issue can be frustrating, but it’s often caused by minor misconfigurations in your pubspec.yaml
file, asset paths, or build cache. By following these quick fixes and solutions, you should be able to resolve any asset issues in your Flutter project. Always remember to check the file structure, rebuild your app after making changes, and verify that asset paths are correct in both the pubspec.yaml
file and your code.
Following these best practices will help ensure that your assets load correctly every time and that your Flutter project runs smoothly.
You can read also: Efficient String Manipulation Techniques Using Substrings In Flutter